core-js
Standard Library
README
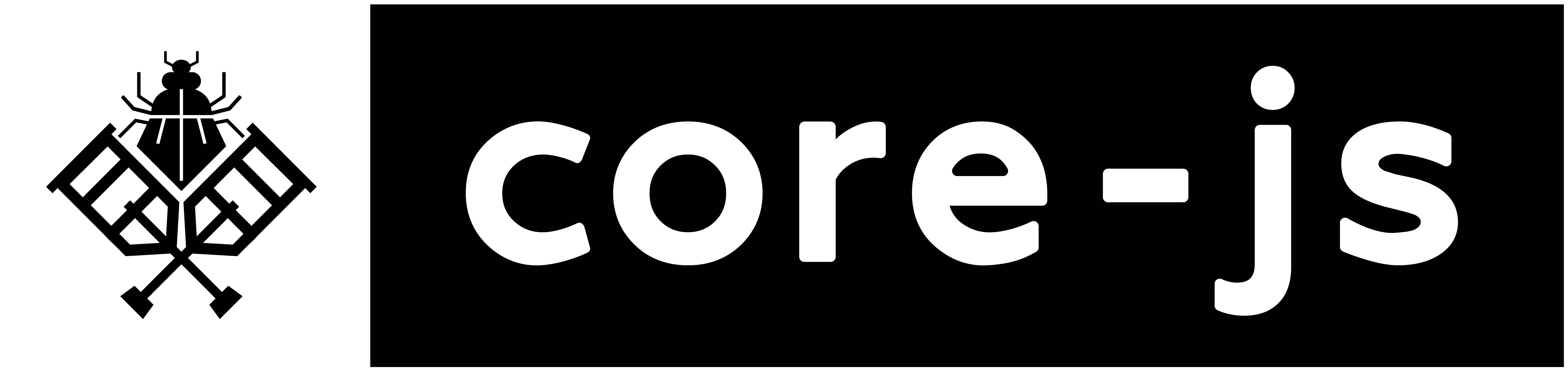
Modular standard library for JavaScript. Includes polyfills for ECMAScript up to 2023: promises, symbols, collections, iterators, typed arrays, many other features, ECMAScript proposals, some cross-platform WHATWG / W3C features and proposals like [URL](#url-and-urlsearchparams). You can load only required features or use it without global namespace pollution.
As advertising: the author is looking for a good job -)
Raising funds
- ``` js
- import 'core-js/actual'; // <- at the top of your entry point
- Array.from(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3]
- [1, 2, 3, 4, 5].group(it => it % 2); // => { 1: [1, 3, 5], 0: [2, 4] }
- Promise.resolve(42).then(x => console.log(x)); // => 42
- structuredClone(new Set([1, 2, 3])); // => new Set([1, 2, 3])
- queueMicrotask(() => console.log('called as microtask'));
- ```
- ``` js
- import 'core-js/actual/array/from'; // <- at the top of your entry point
- import 'core-js/actual/array/group'; // <- at the top of your entry point
- import 'core-js/actual/set'; // <- at the top of your entry point
- import 'core-js/actual/promise'; // <- at the top of your entry point
- import 'core-js/actual/structured-clone'; // <- at the top of your entry point
- import 'core-js/actual/queue-microtask'; // <- at the top of your entry point
- Array.from(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3]
- [1, 2, 3, 4, 5].group(it => it % 2); // => { 1: [1, 3, 5], 0: [2, 4] }
- Promise.resolve(42).then(x => console.log(x)); // => 42
- structuredClone(new Set([1, 2, 3])); // => new Set([1, 2, 3])
- queueMicrotask(() => console.log('called as microtask'));
- ```
- ``` js
- import from from 'core-js-pure/actual/array/from';
- import group from 'core-js-pure/actual/array/group';
- import Set from 'core-js-pure/actual/set';
- import Promise from 'core-js-pure/actual/promise';
- import structuredClone from 'core-js-pure/actual/structured-clone';
- import queueMicrotask from 'core-js-pure/actual/queue-microtask';
- from(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3]
- group([1, 2, 3, 4, 5], it => it % 2); // => { 1: [1, 3, 5], 0: [2, 4] }
- Promise.resolve(42).then(x => console.log(x)); // => 42
- structuredClone(new Set([1, 2, 3])); // => new Set([1, 2, 3])
- queueMicrotask(() => console.log('called as microtask'));
- ```
Index
Usage⬆
Installation:⬆
- ```sh
- // global version
- npm install --save core-js@3.26.1
- // version without global namespace pollution
- npm install --save core-js-pure@3.26.1
- // bundled global version
- npm install --save core-js-bundle@3.26.1
- ```
postinstall message⬆
- ```sh
- ADBLOCK=true npm install
- // or
- DISABLE_OPENCOLLECTIVE=true npm install
- // or
- npm install --loglevel silent
- ```
CommonJS API⬆
- ``` js
- // polyfill all `core-js` features, including early-stage proposals:
- import "core-js";
- // or:
- import "core-js/full";
- // polyfill all actual features - stable ES, web standards and stage 3 ES proposals:
- import "core-js/actual";
- // polyfill only stable features - ES and web standards:
- import "core-js/stable";
- // polyfill only stable ES features:
- import "core-js/es";
- // if you want to polyfill `Set`:
- // all `Set`-related features, with early-stage ES proposals:
- import "core-js/full/set";
- // stable required for `Set` ES features, features from web standards and stage 3 ES proposals:
- import "core-js/actual/set";
- // stable required for `Set` ES features and features from web standards
- // (DOM collections iterator in this case):
- import "core-js/stable/set";
- // only stable ES features required for `Set`:
- import "core-js/es/set";
- // the same without global namespace pollution:
- import Set from "core-js-pure/full/set";
- import Set from "core-js-pure/actual/set";
- import Set from "core-js-pure/stable/set";
- import Set from "core-js-pure/es/set";
- // if you want to polyfill just required methods:
- import "core-js/full/set/intersection";
- import "core-js/actual/array/find-last";
- import "core-js/stable/queue-microtask";
- import "core-js/es/array/from";
- // polyfill iterator helpers proposal:
- import "core-js/proposals/iterator-helpers";
- // polyfill all stage 2+ proposals:
- import "core-js/stage/2";
- ```
Caveats when using CommonJS API:⬆
CommonJS and prototype methods without global namespace pollution⬆
- ``` js
- import fill from 'core-js-pure/actual/array/virtual/fill';
- import findIndex from 'core-js-pure/actual/array/virtual/find-index';
- Array(10)::fill(0).map((a, b) => b * b)::findIndex(it => it && !(it % 8)); // => 4
- ```
Warning! The bind operator is an early-stage ECMAScript proposal and usage of this syntax can be dangerous.
Babel⬆
@babel/polyfill⬆
- ``` js
- import 'core-js/stable';
- import 'regenerator-runtime/runtime';
- ```
@babel/preset-env⬆
Warning! Recommended to specify used minor core-js version, like corejs: '3.26', instead of corejs: 3, since with corejs: 3 will not be injected modules which were added in minor core-js releases.
- ``` js
- import 'core-js/stable';
- ```
- ``` js
- import "core-js/modules/es.array.unscopables.flat";
- import "core-js/modules/es.array.unscopables.flat-map";
- import "core-js/modules/es.object.from-entries";
- import "core-js/modules/web.immediate";
- ```
- ``` js
- import 'core-js/es';
- import 'core-js/proposals/set-methods';
- import 'core-js/full/set/map';
- ```
- ``` js
- import "core-js/modules/es.array.unscopables.flat";
- import "core-js/modules/es.array.unscopables.flat-map";
- import "core-js/modules/es.object.from-entries";
- import "core-js/modules/esnext.set.difference";
- import "core-js/modules/esnext.set.intersection";
- import "core-js/modules/esnext.set.is-disjoint-from";
- import "core-js/modules/esnext.set.is-subset-of";
- import "core-js/modules/esnext.set.is-superset-of";
- import "core-js/modules/esnext.set.map";
- import "core-js/modules/esnext.set.symmetric-difference";
- import "core-js/modules/esnext.set.union";
- ```
- ``` js
- // first file:
- var set = new Set([1, 2, 3]);
- // second file:
- var array = Array.of(1, 2, 3);
- ```
- ``` js
- // first file:
- import 'core-js/modules/es.array.iterator';
- import 'core-js/modules/es.object.to-string';
- import 'core-js/modules/es.set';
- var set = new Set([1, 2, 3]);
- // second file:
- import 'core-js/modules/es.array.of';
- var array = Array.of(1, 2, 3);
- ```
Warning! In the case of useBuiltIns: 'usage', you should not add core-js imports by yourself, they will be added automatically.
@babel/runtime⬆
- ``` js
- import from from 'core-js-pure/stable/array/from';
- import flat from 'core-js-pure/stable/array/flat';
- import Set from 'core-js-pure/stable/set';
- import Promise from 'core-js-pure/stable/promise';
- from(new Set([1, 2, 3, 2, 1]));
- flat([1, [2, 3], [4, [5]]], 2);
- Promise.resolve(32).then(x => console.log(x));
- ```
- ``` js
- Array.from(new Set([1, 2, 3, 2, 1]));
- [1, [2, 3], [4, [5]]].flat(2);
- Promise.resolve(32).then(x => console.log(x));
- ```
Warning! If you use @babel/preset-env and @babel/runtime together, use corejs option only in one place since it's duplicate functionality and will cause conflicts.
swc⬆
- ``` json
- {
- "env": {
- "targets": "> 0.25%, not dead",
- "mode": "entry",
- "coreJs": "3.26"
- }
- }
- ```
Configurable level of aggressiveness⬆
- ``` js
- const configurator = require('core-js/configurator');
- configurator({
- useNative: ['Promise'], // polyfills will be used only if natives completely unavailable
- usePolyfill: ['Array.from', 'String.prototype.padEnd'], // polyfills will be used anyway
- useFeatureDetection: ['Map', 'Set'], // default behaviour
- });
- require('core-js/actual');
- ```
Custom build⬆
Supported engines and compatibility data⬆
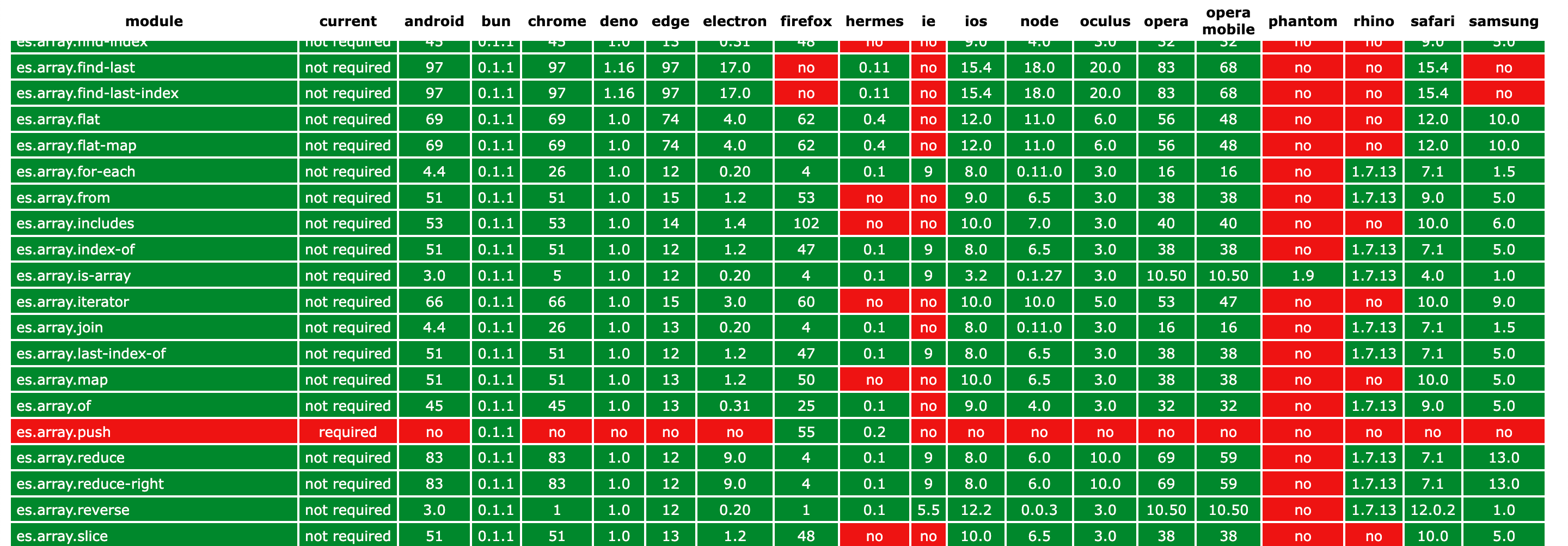
Features:⬆
- ```
- core-js(-pure)
- ```
ECMAScript⬆
- ```
- core-js(-pure)/es
- ```
ECMAScript: Object⬆
- ``` js
- class Object {
- toString(): string; // ES2015+ fix: @@toStringTag support
- __defineGetter__(property: PropertyKey, getter: Function): void;
- __defineSetter__(property: PropertyKey, setter: Function): void;
- __lookupGetter__(property: PropertyKey): Function | void;
- __lookupSetter__(property: PropertyKey): Function | void;
- __proto__: Object | null; // required a way setting of prototype - will not in IE10-, it's for modern engines like Deno
- static assign(target: Object, ...sources: Array<Object>): Object;
- static create(prototype: Object | null, properties?: { [property: PropertyKey]: PropertyDescriptor }): Object;
- static defineProperties(object: Object, properties: { [property: PropertyKey]: PropertyDescriptor })): Object;
- static defineProperty(object: Object, property: PropertyKey, attributes: PropertyDescriptor): Object;
- static entries(object: Object): Array<[string, mixed]>;
- static freeze(object: any): any;
- static fromEntries(iterable: Iterable<[key, value]>): Object;
- static getOwnPropertyDescriptor(object: any, property: PropertyKey): PropertyDescriptor | void;
- static getOwnPropertyDescriptors(object: any): { [property: PropertyKey]: PropertyDescriptor };
- static getOwnPropertyNames(object: any): Array<string>;
- static getPrototypeOf(object: any): Object | null;
- static hasOwn(object: object, key: PropertyKey): boolean;
- static is(value1: any, value2: any): boolean;
- static isExtensible(object: any): boolean;
- static isFrozen(object: any): boolean;
- static isSealed(object: any): boolean;
- static keys(object: any): Array<string>;
- static preventExtensions(object: any): any;
- static seal(object: any): any;
- static setPrototypeOf(target: any, prototype: Object | null): any; // required __proto__ - IE11+
- static values(object: any): Array<mixed>;
- }
- ```
- ```
- core-js(-pure)/es|stable|actual|full/object
- core-js(-pure)/es|stable|actual|full/object/assign
- core-js(-pure)/es|stable|actual|full/object/is
- core-js(-pure)/es|stable|actual|full/object/set-prototype-of
- core-js(-pure)/es|stable|actual|full/object/get-prototype-of
- core-js(-pure)/es|stable|actual|full/object/create
- core-js(-pure)/es|stable|actual|full/object/define-property
- core-js(-pure)/es|stable|actual|full/object/define-properties
- core-js(-pure)/es|stable|actual|full/object/get-own-property-descriptor
- core-js(-pure)/es|stable|actual|full/object/get-own-property-descriptors
- core-js(-pure)/es|stable|actual|full/object/has-own
- core-js(-pure)/es|stable|actual|full/object/keys
- core-js(-pure)/es|stable|actual|full/object/values
- core-js(-pure)/es|stable|actual|full/object/entries
- core-js(-pure)/es|stable|actual|full/object/get-own-property-names
- core-js(-pure)/es|stable|actual|full/object/freeze
- core-js(-pure)/es|stable|actual|full/object/from-entries
- core-js(-pure)/es|stable|actual|full/object/seal
- core-js(-pure)/es|stable|actual|full/object/prevent-extensions
- core-js/es|stable|actual|full/object/proto
- core-js(-pure)/es|stable|actual|full/object/is-frozen
- core-js(-pure)/es|stable|actual|full/object/is-sealed
- core-js(-pure)/es|stable|actual|full/object/is-extensible
- core-js/es|stable|actual|full/object/to-string
- core-js(-pure)/es|stable|actual|full/object/define-getter
- core-js(-pure)/es|stable|actual|full/object/define-setter
- core-js(-pure)/es|stable|actual|full/object/lookup-getter
- core-js(-pure)/es|stable|actual|full/object/lookup-setter
- ```
- ``` js
- let foo = { q: 1, w: 2 };
- let bar = { e: 3, r: 4 };
- let baz = { t: 5, y: 6 };
- Object.assign(foo, bar, baz); // => foo = { q: 1, w: 2, e: 3, r: 4, t: 5, y: 6 }
- Object.is(NaN, NaN); // => true
- Object.is(0, -0); // => false
- Object.is(42, 42); // => true
- Object.is(42, '42'); // => false
- function Parent() {}
- function Child() {}
- Object.setPrototypeOf(Child.prototype, Parent.prototype);
- new Child() instanceof Child; // => true
- new Child() instanceof Parent; // => true
- let object = {
- [Symbol.toStringTag]: 'Foo'
- };
- '' + object; // => '[object Foo]'
- Object.keys('qwe'); // => ['0', '1', '2']
- Object.getPrototypeOf('qwe') === String.prototype; // => true
- Object.values({ a: 1, b: 2, c: 3 }); // => [1, 2, 3]
- Object.entries({ a: 1, b: 2, c: 3 }); // => [['a', 1], ['b', 2], ['c', 3]]
- for (let [key, value] of Object.entries({ a: 1, b: 2, c: 3 })) {
- console.log(key); // => 'a', 'b', 'c'
- console.log(value); // => 1, 2, 3
- }
- // Shallow object cloning with prototype and descriptors:
- let copy = Object.create(Object.getPrototypeOf(object), Object.getOwnPropertyDescriptors(object));
- // Mixin:
- Object.defineProperties(target, Object.getOwnPropertyDescriptors(source));
- const map = new Map([['a', 1], ['b', 2]]);
- Object.fromEntries(map); // => { a: 1, b: 2 }
- class Unit {
- constructor(id) {
- this.id = id;
- }
- toString() {
- return `unit${ this.id }`;
- }
- }
- const units = new Set([new Unit(101), new Unit(102)]);
- Object.fromEntries(units.entries()); // => { unit101: Unit { id: 101 }, unit102: Unit { id: 102 } }
- Object.hasOwn({ foo: 42 }, 'foo'); // => true
- Object.hasOwn({ foo: 42 }, 'bar'); // => false
- Object.hasOwn({}, 'toString'); // => false
- ```
ECMAScript: Function⬆
- ``` js
- class Function {
- name: string;
- bind(thisArg: any, ...args: Array<mixed>): Function;
- @@hasInstance(value: any): boolean;
- }
- ```
- ```
- core-js/es|stable|actual|full/function
- core-js/es|stable|actual|full/function/name
- core-js/es|stable|actual|full/function/has-instance
- core-js(-pure)/es|stable|actual|full/function/bind
- core-js(-pure)/es|stable|actual|full/function/virtual/bind
- ```
- ``` js
- (function foo() {}).name // => 'foo'
- console.log.bind(console, 42)(43); // => 42 43
- ```
ECMAScript: Error⬆
- ``` js
- class [
- Error,
- EvalError,
- RangeError,
- ReferenceError,
- SyntaxError,
- TypeError,
- URIError,
- WebAssembly.CompileError,
- WebAssembly.LinkError,
- WebAssembly.RuntimeError,
- ] {
- constructor(message: string, { cause: any }): %Error%;
- }
- class AggregateError {
- constructor(errors: Iterable, message: string, { cause: any }): AggregateError;
- errors: Array<any>;
- message: string;
- }
- class Error {
- toString(): string; // different fixes
- }
- ```
- ```
- core-js(-pure)/es|stable|actual|full/aggregate-error
- core-js/es|stable|actual|full/error
- core-js/es|stable|actual|full/error/constructor
- core-js/es|stable|actual|full/error/to-string
- ```
- ``` js
- const error1 = new TypeError('Error 1');
- const error2 = new TypeError('Error 2');
- const aggregate = new AggregateError([error1, error2], 'Collected errors');
- aggregate.errors[0] === error1; // => true
- aggregate.errors[1] === error2; // => true
- const cause = new TypeError('Something wrong');
- const error = new TypeError('Here explained what`s wrong', { cause });
- error.cause === cause; // => true
- Error.prototype.toString.call({ message: 1, name: 2 }) === '2: 1'; // => true
- ```
ECMAScript: Array⬆
- ``` js
- class Array {
- at(index: int): any;
- concat(...args: Array<mixed>): Array<mixed>; // with adding support of @@isConcatSpreadable and @@species
- copyWithin(target: number, start: number, end?: number): this;
- entries(): Iterator<[index, value]>;
- every(callbackfn: (value: any, index: number, target: any) => boolean, thisArg?: any): boolean;
- fill(value: any, start?: number, end?: number): this;
- filter(callbackfn: (value: any, index: number, target: any) => boolean, thisArg?: any): Array<mixed>; // with adding support of @@species
- find(callbackfn: (value: any, index: number, target: any) => boolean), thisArg?: any): any;
- findIndex(callbackfn: (value: any, index: number, target: any) => boolean, thisArg?: any): uint;
- findLast(callbackfn: (value: any, index: number, target: any) => boolean, thisArg?: any): any;
- findLastIndex(callbackfn: (value: any, index: number, target: any) => boolean, thisArg?: any): uint;
- flat(depthArg?: number = 1): Array<mixed>;
- flatMap(mapFn: (value: any, index: number, target: any) => any, thisArg: any): Array<mixed>;
- forEach(callbackfn: (value: any, index: number, target: any) => void, thisArg?: any): void;
- includes(searchElement: any, from?: number): boolean;
- indexOf(searchElement: any, from?: number): number;
- join(separator: string = ','): string;
- keys(): Iterator<index>;
- lastIndexOf(searchElement: any, from?: number): number;
- map(mapFn: (value: any, index: number, target: any) => any, thisArg?: any): Array<mixed>; // with adding support of @@species
- push(...args: Array<mixed>): uint;
- reduce(callbackfn: (memo: any, value: any, index: number, target: any) => any, initialValue?: any): any;
- reduceRight(callbackfn: (memo: any, value: any, index: number, target: any) => any, initialValue?: any): any;
- reverse(): this; // Safari 12.0 bug fix
- slice(start?: number, end?: number): Array<mixed>; // with adding support of @@species
- splice(start?: number, deleteCount?: number, ...items: Array<mixed>): Array<mixed>; // with adding support of @@species
- some(callbackfn: (value: any, index: number, target: any) => boolean, thisArg?: any): boolean;
- sort(comparefn?: (a: any, b: any) => number): this; // with modern behavior like stable sort
- unshift(...args: Array<mixed>): uint;
- values(): Iterator<value>;
- @@iterator(): Iterator<value>;
- @@unscopables: { [newMethodNames: string]: true };
- static from(items: Iterable | ArrayLike, mapFn?: (value: any, index: number) => any, thisArg?: any): Array<mixed>;
- static isArray(value: any): boolean;
- static of(...args: Array<mixed>): Array<mixed>;
- }
- class Arguments {
- @@iterator(): Iterator<value>; // available only in core-js methods
- }
- ```
- ```
- core-js(-pure)/es|stable|actual|full/array
- core-js(-pure)/es|stable|actual|full/array/from
- core-js(-pure)/es|stable|actual|full/array/of
- core-js(-pure)/es|stable|actual|full/array/is-array
- core-js(-pure)/es|stable|actual|full/array/at
- core-js(-pure)/es|stable|actual|full/array/concat
- core-js(-pure)/es|stable|actual|full/array/entries
- core-js(-pure)/es|stable|actual|full/array/every
- core-js(-pure)/es|stable|actual|full/array/copy-within
- core-js(-pure)/es|stable|actual|full/array/fill
- core-js(-pure)/es|stable|actual|full/array/filter
- core-js(-pure)/es|stable|actual|full/array/find
- core-js(-pure)/es|stable|actual|full/array/find-index
- core-js(-pure)/es|stable|actual|full/array/find-last
- core-js(-pure)/es|stable|actual|full/array/find-last-index
- core-js(-pure)/es|stable|actual|full/array/flat
- core-js(-pure)/es|stable|actual|full/array/flat-map
- core-js(-pure)/es|stable|actual|full/array/for-each
- core-js(-pure)/es|stable|actual|full/array/includes
- core-js(-pure)/es|stable|actual|full/array/index-of
- core-js(-pure)/es|stable|actual|full/array/iterator
- core-js(-pure)/es|stable|actual|full/array/join
- core-js(-pure)/es|stable|actual|full/array/keys
- core-js(-pure)/es|stable|actual|full/array/last-index-of
- core-js(-pure)/es|stable|actual|full/array/map
- core-js(-pure)/es|stable|actual|full/array/push
- core-js(-pure)/es|stable|actual|full/array/reduce
- core-js(-pure)/es|stable|actual|full/array/reduce-right
- core-js(-pure)/es|stable|actual|full/array/reverse
- core-js(-pure)/es|stable|actual|full/array/slice
- core-js(-pure)/es|stable|actual|full/array/splice
- core-js(-pure)/es|stable|actual|full/array/some
- core-js(-pure)/es|stable|actual|full/array/sort
- core-js(-pure)/es|stable|actual|full/array/unshift
- core-js(-pure)/es|stable|actual|full/array/values
- core-js(-pure)/es|stable|actual|full/array/virtual/at
- core-js(-pure)/es|stable|actual|full/array/virtual/concat
- core-js(-pure)/es|stable|actual|full/array/virtual/copy-within
- core-js(-pure)/es|stable|actual|full/array/virtual/entries
- core-js(-pure)/es|stable|actual|full/array/virtual/every
- core-js(-pure)/es|stable|actual|full/array/virtual/fill
- core-js(-pure)/es|stable|actual|full/array/virtual/filter
- core-js(-pure)/es|stable|actual|full/array/virtual/find
- core-js(-pure)/es|stable|actual|full/array/virtual/find-index
- core-js(-pure)/es|stable|actual|full/array/virtual/find-last
- core-js(-pure)/es|stable|actual|full/array/virtual/find-last-index
- core-js(-pure)/es|stable|actual|full/array/virtual/flat
- core-js(-pure)/es|stable|actual|full/array/virtual/flat-map
- core-js(-pure)/es|stable|actual|full/array/virtual/for-each
- core-js(-pure)/es|stable|actual|full/array/virtual/includes
- core-js(-pure)/es|stable|actual|full/array/virtual/index-of
- core-js(-pure)/es|stable|actual|full/array/virtual/iterator
- core-js(-pure)/es|stable|actual|full/array/virtual/join
- core-js(-pure)/es|stable|actual|full/array/virtual/keys
- core-js(-pure)/es|stable|actual|full/array/virtual/last-index-of
- core-js(-pure)/es|stable|actual|full/array/virtual/map
- core-js(-pure)/es|stable|actual|full/array/virtual/push
- core-js(-pure)/es|stable|actual|full/array/virtual/reduce
- core-js(-pure)/es|stable|actual|full/array/virtual/reduce-right
- core-js(-pure)/es|stable|actual|full/array/virtual/reverse
- core-js(-pure)/es|stable|actual|full/array/virtual/slice
- core-js(-pure)/es|stable|actual|full/array/virtual/some
- core-js(-pure)/es|stable|actual|full/array/virtual/sort
- core-js(-pure)/es|stable|actual|full/array/virtual/splice
- core-js(-pure)/es|stable|actual|full/array/virtual/unshift
- core-js(-pure)/es|stable|actual|full/array/virtual/values
- ```
- ``` js
- Array.from(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3]
- Array.from({ 0: 1, 1: 2, 2: 3, length: 3 }); // => [1, 2, 3]
- Array.from('123', Number); // => [1, 2, 3]
- Array.from('123', it => it * it); // => [1, 4, 9]
- Array.of(1); // => [1]
- Array.of(1, 2, 3); // => [1, 2, 3]
- let array = ['a', 'b', 'c'];
- for (let value of array) console.log(value); // => 'a', 'b', 'c'
- for (let value of array.values()) console.log(value); // => 'a', 'b', 'c'
- for (let key of array.keys()) console.log(key); // => 0, 1, 2
- for (let [key, value] of array.entries()) {
- console.log(key); // => 0, 1, 2
- console.log(value); // => 'a', 'b', 'c'
- }
- function isOdd(value) {
- return value % 2;
- }
- [4, 8, 15, 16, 23, 42].find(isOdd); // => 15
- [4, 8, 15, 16, 23, 42].findIndex(isOdd); // => 2
- [1, 2, 3, 4].findLast(isOdd); // => 3
- [1, 2, 3, 4].findLastIndex(isOdd); // => 2
- Array(5).fill(42); // => [42, 42, 42, 42, 42]
- [1, 2, 3, 4, 5].copyWithin(0, 3); // => [4, 5, 3, 4, 5]
- [1, 2, 3].includes(2); // => true
- [1, 2, 3].includes(4); // => false
- [1, 2, 3].includes(2, 2); // => false
- [NaN].indexOf(NaN); // => -1
- [NaN].includes(NaN); // => true
- Array(1).indexOf(undefined); // => -1
- Array(1).includes(undefined); // => true
- [1, [2, 3], [4, 5]].flat(); // => [1, 2, 3, 4, 5]
- [1, [2, [3, [4]]], 5].flat(); // => [1, 2, [3, [4]], 5]
- [1, [2, [3, [4]]], 5].flat(3); // => [1, 2, 3, 4, 5]
- [{ a: 1, b: 2 }, { a: 3, b: 4 }, { a: 5, b: 6 }].flatMap(it => [it.a, it.b]); // => [1, 2, 3, 4, 5, 6]
- [1, 2, 3].at(1); // => 2
- [1, 2, 3].at(-1); // => 3
- ```
ECMAScript: String and RegExp⬆
- ``` js
- class String {
- static fromCodePoint(...codePoints: Array<number>): string;
- static raw({ raw: Array<string> }, ...substitutions: Array<string>): string;
- at(index: int): string;
- includes(searchString: string, position?: number): boolean;
- startsWith(searchString: string, position?: number): boolean;
- endsWith(searchString: string, position?: number): boolean;
- repeat(count: number): string;
- padStart(length: number, fillStr?: string = ' '): string;
- padEnd(length: number, fillStr?: string = ' '): string;
- codePointAt(pos: number): number | void;
- match(template: any): any; // ES2015+ fix for support @@match
- matchAll(regexp: RegExp): Iterator;
- replace(template: any, replacer: any): any; // ES2015+ fix for support @@replace
- replaceAll(searchValue: string | RegExp, replaceString: string | (searchValue, index, this) => string): string;
- search(template: any): any; // ES2015+ fix for support @@search
- split(template: any, limit?: int): Array<string>;; // ES2015+ fix for support @@split, some fixes for old engines
- trim(): string;
- trimLeft(): string;
- trimRight(): string;
- trimStart(): string;
- trimEnd(): string;
- anchor(name: string): string;
- big(): string;
- blink(): string;
- bold(): string;
- fixed(): string;
- fontcolor(color: string): string;
- fontsize(size: any): string;
- italics(): string;
- link(url: string): string;
- small(): string;
- strike(): string;
- sub(): string;
- substr(start: int, length?: int): string;
- sup(): string;
- @@iterator(): Iterator<characters>;
- }
- class RegExp {
- // support of sticky (`y`) flag, dotAll (`s`) flag, named capture groups, can alter flags
- constructor(pattern: RegExp | string, flags?: string): RegExp;
- exec(): Array<string | undefined> | null; // IE8 fixes
- test(string: string): boolean; // delegation to `.exec`
- toString(): string; // ES2015+ fix - generic
- @@match(string: string): Array | null;
- @@matchAll(string: string): Iterator;
- @@replace(string: string, replaceValue: Function | string): string;
- @@search(string: string): number;
- @@split(string: string, limit: number): Array<string>;
- readonly attribute dotAll: boolean; // IE9+
- readonly attribute flags: string; // IE9+
- readonly attribute sticky: boolean; // IE9+
- }
- function escape(string: string): string;
- function unescape(string: string): string;
- ```
- ```
- core-js(-pure)/es|stable|actual|full/string
- core-js(-pure)/es|stable|actual|full/string/from-code-point
- core-js(-pure)/es|stable|actual|full/string/raw
- core-js/es|stable|actual|full/string/match
- core-js/es|stable|actual|full/string/replace
- core-js/es|stable|actual|full/string/search
- core-js/es|stable|actual|full/string/split
- core-js(-pure)/es|stable|actual/string(/virtual)/at
- core-js(-pure)/es|stable|actual|full/string(/virtual)/code-point-at
- core-js(-pure)/es|stable|actual|full/string(/virtual)/ends-with
- core-js(-pure)/es|stable|actual|full/string(/virtual)/includes
- core-js(-pure)/es|stable|actual|full/string(/virtual)/starts-with
- core-js(-pure)/es|stable|actual|full/string(/virtual)/match-all
- core-js(-pure)/es|stable|actual|full/string(/virtual)/pad-start
- core-js(-pure)/es|stable|actual|full/string(/virtual)/pad-end
- core-js(-pure)/es|stable|actual|full/string(/virtual)/repeat
- core-js(-pure)/es|stable|actual|full/string(/virtual)/replace-all
- core-js(-pure)/es|stable|actual|full/string(/virtual)/trim
- core-js(-pure)/es|stable|actual|full/string(/virtual)/trim-start
- core-js(-pure)/es|stable|actual|full/string(/virtual)/trim-end
- core-js(-pure)/es|stable|actual|full/string(/virtual)/trim-left
- core-js(-pure)/es|stable|actual|full/string(/virtual)/trim-right
- core-js(-pure)/es|stable|actual|full/string(/virtual)/anchor
- core-js(-pure)/es|stable|actual|full/string(/virtual)/big
- core-js(-pure)/es|stable|actual|full/string(/virtual)/blink
- core-js(-pure)/es|stable|actual|full/string(/virtual)/bold
- core-js(-pure)/es|stable|actual|full/string(/virtual)/fixed
- core-js(-pure)/es|stable|actual|full/string(/virtual)/fontcolor
- core-js(-pure)/es|stable|actual|full/string(/virtual)/fontsize
- core-js(-pure)/es|stable|actual|full/string(/virtual)/italics
- core-js(-pure)/es|stable|actual|full/string(/virtual)/link
- core-js(-pure)/es|stable|actual|full/string(/virtual)/small
- core-js(-pure)/es|stable|actual|full/string(/virtual)/strike
- core-js(-pure)/es|stable|actual|full/string(/virtual)/sub
- core-js(-pure)/es|stable|actual|full/string(/virtual)/substr
- core-js(-pure)/es|stable|actual|full/string(/virtual)/sup
- core-js(-pure)/es|stable|actual|full/string(/virtual)/iterator
- core-js/es|stable|actual|full/regexp
- core-js/es|stable|actual|full/regexp/constructor
- core-js/es|stable|actual|full/regexp/dot-all
- core-js(-pure)/es|stable|actual|full/regexp/flags
- core-js/es|stable|actual|full/regexp/sticky
- core-js/es|stable|actual|full/regexp/test
- core-js/es|stable|actual|full/regexp/to-string
- core-js/es|stable|actual|full/escape
- core-js/es|stable|actual|full/unescape
- ```
- ``` js
- for (let value of 'a𠮷b') {
- console.log(value); // => 'a', '𠮷', 'b'
- }
- 'foobarbaz'.includes('bar'); // => true
- 'foobarbaz'.includes('bar', 4); // => false
- 'foobarbaz'.startsWith('foo'); // => true
- 'foobarbaz'.startsWith('bar', 3); // => true
- 'foobarbaz'.endsWith('baz'); // => true
- 'foobarbaz'.endsWith('bar', 6); // => true
- 'string'.repeat(3); // => 'stringstringstring'
- 'hello'.padStart(10); // => ' hello'
- 'hello'.padStart(10, '1234'); // => '12341hello'
- 'hello'.padEnd(10); // => 'hello '
- 'hello'.padEnd(10, '1234'); // => 'hello12341'
- '𠮷'.codePointAt(0); // => 134071
- String.fromCodePoint(97, 134071, 98); // => 'a𠮷b'
- let name = 'Bob';
- String.raw`Hi\n${name}!`; // => 'Hi\\nBob!' (ES2015 template string syntax)
- String.raw({ raw: 'test' }, 0, 1, 2); // => 't0e1s2t'
- 'foo'.bold(); // => 'foo'
- 'bar'.anchor('a"b'); // => 'bar'
- 'baz'.link('https://example.com'); // => 'baz'
- RegExp('.', 's').test('\n'); // => true
- RegExp('.', 's').dotAll; // => true
- RegExp('foo:(?<foo>\\w+),bar:(?<bar>\\w+)').exec('foo:abc,bar:def').groups.bar; // => 'def'
- 'foo:abc,bar:def'.replace(RegExp('foo:(?<foo>\\w+),bar:(?<bar>\\w+)'), '$<bar>,$<foo>'); // => 'def,abc'
- RegExp(/./g, 'm'); // => /./m
- /foo/.flags; // => ''
- /foo/gim.flags; // => 'gim'
- RegExp('foo', 'y').sticky; // => true
- const text = 'First line\nSecond line';
- const regex = RegExp('(\\S+) line\\n?', 'y');
- regex.exec(text)[1]; // => 'First'
- regex.exec(text)[1]; // => 'Second'
- regex.exec(text); // => null
- 'foo'.match({ [Symbol.match]: () => 1 }); // => 1
- 'foo'.replace({ [Symbol.replace]: () => 2 }); // => 2
- 'foo'.search({ [Symbol.search]: () => 3 }); // => 3
- 'foo'.split({ [Symbol.split]: () => 4 }); // => 4
- RegExp.prototype.toString.call({ source: 'foo', flags: 'bar' }); // => '/foo/bar'
- ' hello '.trimLeft(); // => 'hello '
- ' hello '.trimRight(); // => ' hello'
- ' hello '.trimStart(); // => 'hello '
- ' hello '.trimEnd(); // => ' hello'
- for (let [_, d, D] of '1111a2b3cccc'.matchAll(/(\d)(\D)/g)) {
- console.log(d, D); // => 1 a, 2 b, 3 c
- }
- 'Test abc test test abc test.'.replaceAll('abc', 'foo'); // -> 'Test foo test test foo test.'
- 'abc'.at(1); // => 'b'
- 'abc'.at(-1); // => 'c'
- ```