Dioma
Elegant dependency injection container for vanilla JavaScript and TypeScrip...
README
Dioma
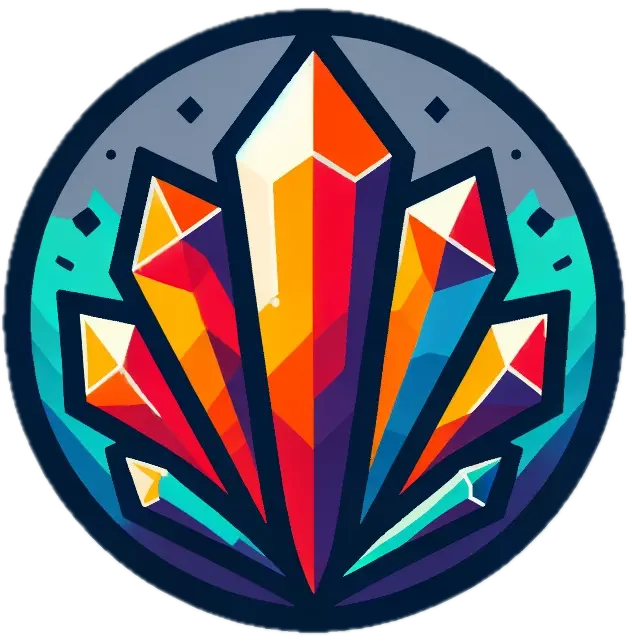
Elegant dependency injection container for vanilla JavaScript and TypeScript
Features
Installation
- ```sh
- npm install --save dioma
- yarn add dioma
- ```
Usage
To start injecting dependencies, you just need to add the static scope property to your class and use the inject function to get the instance of it. By default, inject makes classes "stick" to the container where they were first injected (more details in the Class registration section).
Here's an example of using it for Singleton and Transient scopes:
- ```typescript
- import { inject, Scopes } from "dioma";
- class Garage {
- open() {
- console.log("garage opened");
- }
- // Single instance of the class for the entire application
- static scope = Scopes.Singleton();
- }
- class Car {
- // injects instance of Garage
- constructor(private garage = inject(Garage)) {}
- park() {
- this.garage.open();
- console.log("car parked");
- }
- // New instance of the class on every injection
- static scope = Scopes.Transient();
- }
- // Creates a new Car and injects Garage
- const car = inject(Car);
- car.park();
- ```
Scopes
Dioma supports the following scopes:
- Scopes.Singleton() - creates a single instance of the class
- Scopes.Transient() - creates a new instance of the class on every injection
- Scopes.Container() - creates a single instance of the class per container
- Scopes.Resolution() - creates a new instance of the class every time, but the instance is the same for the entire resolution
- Scopes.Scoped() is the same as Scopes.Container()
Singleton scope
Singleton scope creates a single instance of the class for the entire application.
The instances are stored in the global container, so anyone can access them.
If you want to isolate the class to a specific container, use the Container scope.
A simple example you can see in the Usage section.
Multiple singletons can be cross-referenced with each other using async injection.
Transient scope
Transient scope creates a new instance of the class on every injection:
- ```typescript
- import { inject, Scopes } from "dioma";
- class Engine {
- start() {
- console.log("Engine started");
- }
- static scope = Scopes.Singleton();
- }
- class Vehicle {
- constructor(private engine = inject(Engine)) {}
- drive() {
- this.engine.start();
- console.log("Vehicle driving");
- }
- static scope = Scopes.Transient();
- }
- // New vehicle every time
- const vehicle = inject(Vehicle);
- vehicle.drive();
- ```
Generally, transient scope instances can't be cross-referenced by the async injection with some exceptions.
Container scope
Container scope creates a single instance of the class per container. It's the same as the singleton, but relative to the custom container.
The usage is the same as for the singleton scope, but you need to create a container first and use container.inject instead of inject:
- ```typescript
- import { Container, Scopes } from "dioma";
- const container = new Container();
- class Garage {
- open() {
- console.log("garage opened");
- }
- // Single instance of the class for the container
- static scope = Scopes.Container();
- }
- // Register Garage on the container
- container.register({ class: Garage });
- class Car {
- // Use inject method of the container for Garage
- constructor(private garage = container.inject(Garage)) {}
- park() {
- this.garage.open();
- console.log("car parked");
- }
- // New instance on every injection
- static scope = Scopes.Transient();
- }
- const car = container.inject(Car);
- car.park();
- ```
Container-scoped classes usually are registered in the container first. Without it, the class will "stick" to the container it's used in.