resvg-js
A high-performance SVG renderer and toolkit, powered by Rust based resvg an...
README
resvg-js
Please use all lowercase resvg-js when referencing project names.
💖 🙏 Sponsoring me
Your sponsorship means a lot to me. It will help me sustain my projects actively and make more of my ideas come true. Much appreciated!
Features
- Fast, safe and zero dependencies, with correct output.
- Convert SVG to PNG, includes cropping, scaling and setting the background color.
- Support system fonts and custom fonts in SVG text.
- v2: Gets the width and height of the SVG and the generated PNG.
- `v2`: Support for outputting simplified SVG strings, such as converting shapes(rect, circle, etc) to `- v2: Support WebAssembly.
- v2: Support to get SVG bounding box and crop according to bounding box.
- `v2`: Support for loading images of external links in `- No need for node-gyp and postinstall, the .node file has been compiled for you.
- Cross-platform support, including Apple M Chips.
- Support for running as native addons in Deno.
Sponsor
Alipay(支付宝)
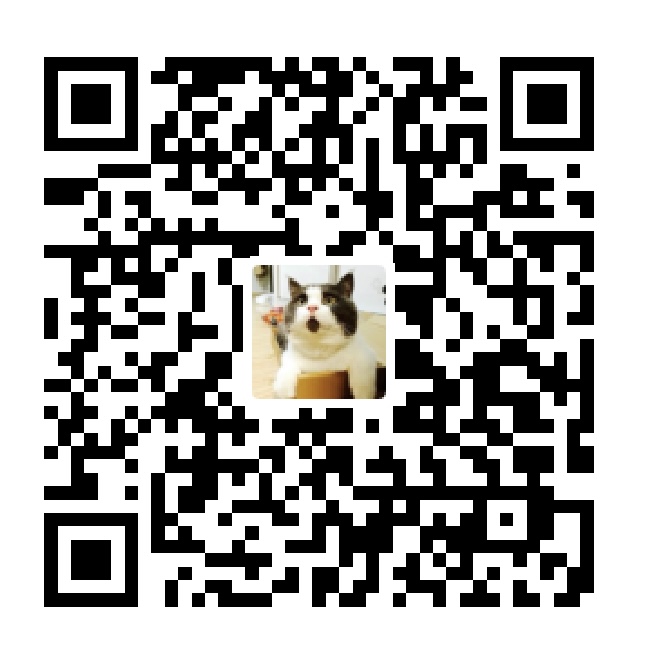
Installation
Node.js
- ```shell
- npm i @resvg/resvg-js
- ```
Browser(Wasm)
- ```html
- <script src="https://unpkg.com/@resvg/resvg-wasm"></script>
- ```
Example
Node.js Example
This example will load Source Han Serif, and then render the SVG to PNG.
- ```shell
- node example/index.js
- Loaded 1 font faces in 0ms.
- Font './example/SourceHanSerifCN-Light-subset.ttf':0 found in 0.006ms.
- ✨ Done in 55.65491008758545 ms
- ```
Deno Example
- ```shell
- deno run --unstable --allow-read --allow-write --allow-ffi example/index-deno.js
- [2022-11-16T15:03:29Z DEBUG resvg_js::fonts] Loaded 1 font faces in 0.067ms.
- [2022-11-16T15:03:29Z DEBUG resvg_js::fonts] Font './example/SourceHanSerifCN-Light-subset.ttf':0 found in 0.001ms.
- Original SVG Size: 1324 x 687
- Output PNG Size : 1200 x 623
- ✨ Done in 66 ms
- ```
Usage
Node.js
- ```js
- const { promises } = require('fs')
- const { join } = require('path')
- const { Resvg } = require('@resvg/resvg-js')
- async function main() {
- const svg = await promises.readFile(join(__dirname, './text.svg'))
- const opts = {
- background: 'rgba(238, 235, 230, .9)',
- fitTo: {
- mode: 'width',
- value: 1200,
- },
- font: {
- fontFiles: ['./example/SourceHanSerifCN-Light-subset.ttf'], // Load custom fonts.
- loadSystemFonts: false, // It will be faster to disable loading system fonts.
- // defaultFontFamily: 'Source Han Serif CN Light', // You can omit this.
- },
- }
- const resvg = new Resvg(svg, opts)
- const pngData = resvg.render()
- const pngBuffer = pngData.asPng()
- console.info('Original SVG Size:', `${resvg.width} x ${resvg.height}`)
- console.info('Output PNG Size :', `${pngData.width} x ${pngData.height}`)
- await promises.writeFile(join(__dirname, './text-out.png'), pngBuffer)
- }
- main()
- ```
Bun
Starting with Bun 0.8.1, resvg-js can be run directly in Bun without any modification to the JS files, and is fully compatible with the syntax in Node.js.
- ```shell
- bun example/index.js
- ```
Deno
Starting with Deno 1.26.1, there is support for running Native Addons directly from Node.js.
This allows for performance that is close to that found in Node.js.
- ```shell
- deno run --unstable --allow-read --allow-write --allow-ffi example/index-deno.js
- ```
- ```js
- import * as path from 'https://deno.land/std@0.159.0/path/mod.ts'
- import { Resvg } from 'npm:@resvg/resvg-js'
- const __dirname = path.dirname(path.fromFileUrl(import.meta.url))
- const svg = await Deno.readFile(path.join(__dirname, './text.svg'))
- const opts = {
- fitTo: {
- mode: 'width',
- value: 1200,
- },
- }
- const t = performance.now()
- const resvg = new Resvg(svg, opts)
- const pngData = resvg.render()
- const pngBuffer = pngData.asPng()
- console.info('Original SVG Size:', `${resvg.width} x ${resvg.height}`)
- console.info('Output PNG Size :', `${pngData.width} x ${pngData.height}`)
- console.info('✨ Done in', performance.now() - t, 'ms')
- await Deno.writeFile(path.join(__dirname, './text-out-deno.png'), pngBuffer)
- ```
WebAssembly
This package also ships a pure WebAssembly artifact built with wasm-bindgen to run in browsers.
Browser
- ```html
- <script src="https://unpkg.com/@resvg/resvg-wasm"></script>
- <script>
- ;(async function () {
- // The Wasm must be initialized first
- await resvg.initWasm(fetch('https://unpkg.com/@resvg/resvg-wasm/index_bg.wasm'))
- const font = await fetch('./fonts/Pacifico-Regular.woff2')
- if (!font.ok) return
- const fontData = await font.arrayBuffer()
- const buffer = new Uint8Array(fontData)
- const opts = {
- fitTo: {
- mode: 'width', // If you need to change the size
- value: 800,
- },
- font: {
- fontBuffers: [buffer], // New in 2.5.0, loading custom fonts
- },
- }
- const svg = '<svg> ... </svg>' // Input SVG, String or Uint8Array
- const resvgJS = new resvg.Resvg(svg, opts)
- const pngData = resvgJS.render(svg, opts) // Output PNG data, Uint8Array
- const pngBuffer = pngData.asPng()
- const svgURL = URL.createObjectURL(new Blob([pngData], { type: 'image/png' }))
- document.getElementById('output').src = svgURL
- })()
- </script>
- ```