React Calendar
Ultimate calendar for your React app.
README
React-Calendar
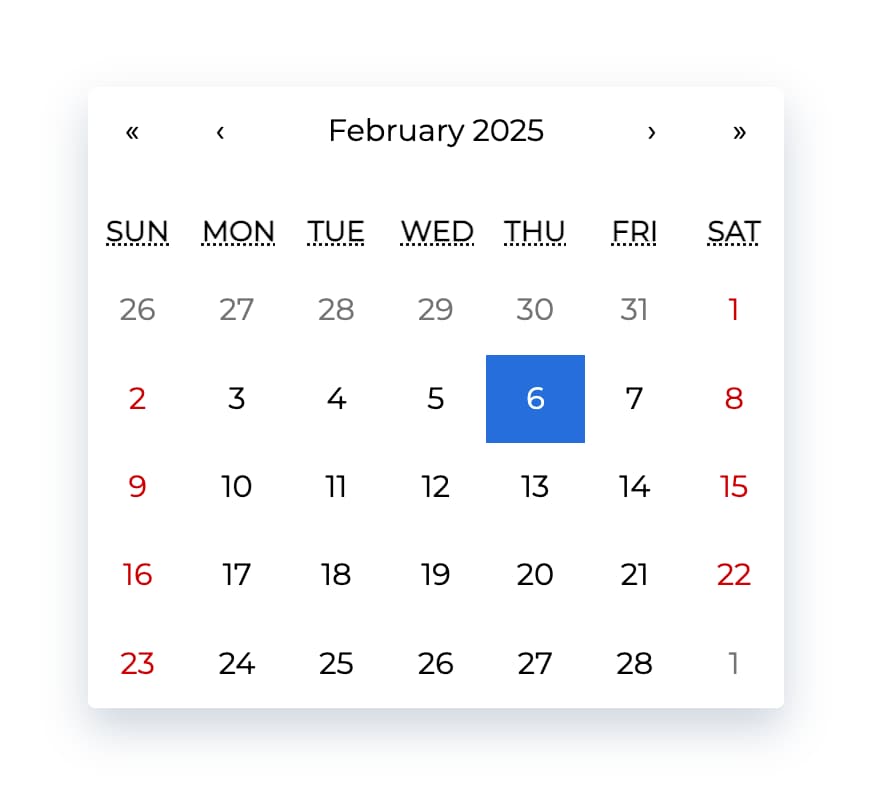
Ultimate calendar for your React app.
- Pick days, months, years, or even decades
- Supports range selection
- Supports virtually any language
- No moment.js needed
tl;dr
- Install by executing npm install react-calendar or yarn add react-calendar.
- Import by adding import Calendar from 'react-calendar'.
- Use by adding `Demo
A minimal demo page can be found in sample directory.
Online demo is also available!
Before you continue
React-Calendar is under constant development. This documentation is written for React-Calendar 3.x branch. If you want to see documentation for other versions of React-Calendar, use dropdown on top of GitHub page to switch to an appropriate tag. Here are quick links to the newest docs from each branch:
- v2.x
Getting started
Compatibility
Your project needs to use React 16.3 or later.
React-Calendar uses modern web technologies. That's why it's so fast, lightweight and easy to style. This, however, comes at a cost of supporting only modern browsers.
Legacy browsers
If you need to support legacy browsers like Internet Explorer 10, you will need to use Intl.js or another Intl polyfill along with React-Calendar.
My locale isn't supported! What can I do?
If your locale isn't supported, you can use Intl.js or another Intl polyfill along with React-Calendar.
Installation
Add React-Calendar to your project by executing npm install react-calendar or yarn add react-calendar.
Usage
Here's an example of basic usage:
- ``` js
- import React, { useState } from 'react';
- import Calendar from 'react-calendar';
- function MyApp() {
- const [value, onChange] = useState(new Date());
- return (
- <div>
- <Calendar onChange={onChange} value={value} />
- </div>
- );
- }
- ```
Check the sample directory in this repository for a full working example. For more examples and more advanced use cases, check Recipes in React-Calendar Wiki.
Custom styling
If you want to use default React-Calendar styling to build upon it, you can import React-Calendar's styles by using:
- ``` js
- import 'react-calendar/dist/Calendar.css';
- ```
User guide
Calendar
Displays a complete, interactive calendar.
Props
Prop | Description | Default | Example |
---|---|---|---|
--- | --- | --- | --- |
activeStartDate | The | (today) | `new |
allowPartialRange | Whether | `false` | `true` |
calendarType | Type | Type | `"ISO |
className | Class | n/a |
|
defaultActiveStartDate | The | (today) | `new |
defaultValue | Calendar | n/a |
|
defaultView | Determines | The | `"year"` |
formatDay | Function | (default | `(locale, |
formatLongDate | Function | (default | `(locale, |
formatMonth | Function | (default | `(locale, |
formatMonthYear | Function | (default | `(locale, |
formatShortWeekday | Function | (default | `(locale, |
formatWeekday | Function | (default | `(locale, |
formatYear | Function | (default | `(locale, |
goToRangeStartOnSelect | Whether | `true` | `false` |
inputRef | A | n/a |
|
locale | Locale | User's | `"hu-HU"` |
maxDate | Maximum | n/a | Date: |
maxDetail | The | `"month"` | `"year"` |
minDate | Minimum | n/a | Date: |
minDetail | The | `"century"` | `"decade"` |
navigationAriaLabel | `aria-label` | n/a | `"Go |
navigationAriaLive | `aria-live` | `undefined` | `"polite"` |
navigationLabel | Content | (default | `` |
nextAriaLabel | `aria-label` | n/a | `"Next"` |
nextLabel | Content | `"›"` |
|
next2AriaLabel | `aria-label` | n/a | `"Jump |
next2Label | Content | `"»"` |
|
onActiveStartDateChange | Function | n/a | `({ |
onChange | Function | n/a | `(value, |
onViewChange | Function | n/a | `({ |
onClickDay | Function | n/a | `(value, |
onClickDecade | Function | n/a | `(value, |
onClickMonth | Function | n/a | `(value, |
onClickWeekNumber | Function | n/a | `(weekNumber, |
onClickYear | Function | n/a | `(value, |
onDrillDown | Function | n/a | `({ |
onDrillUp | Function | n/a | `({ |
prevAriaLabel | `aria-label` | n/a | `"Previous"` |
prevLabel | Content | `"‹"` |
|
prev2AriaLabel | `aria-label` | n/a | `"Jump |
prev2Label | Content | `"«"` |
|
returnValue | Which | `"start"` | `"range"` |
showDoubleView | Whether | `false` | `true` |
showFixedNumberOfWeeks | Whether | `false` | `true` |
showNavigation | Whether | `true` | `false` |
showNeighboringMonth | Whether | `true` | `false` |
selectRange | Whether | `false` | `true` |
showWeekNumbers | Whether | `false` | `true` |
tileClassName | Class | n/a |
|
tileContent | Allows | n/a |
|
tileDisabled | Pass | n/a |
|
value | Calendar | n/a |
|
view | Determines | The | `"year"` |
MonthView, YearView, DecadeView, CenturyView
Displays a given month, year, decade and a century, respectively.
Props
Prop | Description | Default | Example |
---|---|---|---|
--- | --- | --- | --- |
activeStartDate | The | n/a | `new |
hover | The | n/a | `new |
maxDate | Maximum | n/a | Date: |
minDate | Minimum | n/a | Date: |
onClick | Function | n/a | `(value) |
tileClassName | Class | n/a |
|
tileContent | Allows | n/a | `({ |
value | Calendar | n/a |
|
Useful links
License
The MIT License.
Author
![]() | Wojciech Maj kontakt@wojtekmaj.pl https://wojtekmaj.pl |
Thank you
Sponsors
Thank you to all our sponsors! Become a sponsor and get your image on our README on GitHub.
Backers
Thank you to all our backers! Become a backer and get your image on our README on GitHub.
Top Contributors
Thank you to all our contributors that helped on this project!