TypewriterJS
A simple yet powerful native javascript plugin for a cool typewriter effect...
README
TypewriterJS v2
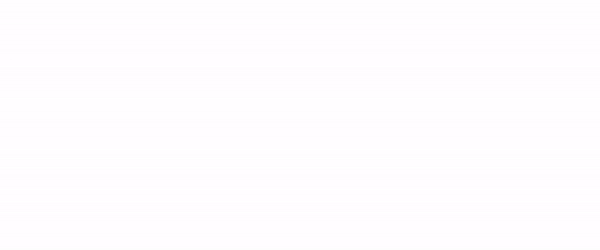
CDN
You can use the CDN version of this plugin for fast and easy setup.
- ```html
- <script src="https://unpkg.com/typewriter-effect@latest/dist/core.js"></script>
- ```
Installation
You can install Typewriterjs with just one command and you're good to go
- ```shell
- # with npm
- npm i typewriter-effect
- # with yarn
- yarn add typewriter-effect
- ```
Core
This include the core typewriter library, which can be used directly through the API.
See examples in the 'examples' folder.
- ```js
- import Typewriter from 'typewriter-effect/dist/core';
- new Typewriter('#typewriter', {
- strings: ['Hello', 'World'],
- autoStart: true,
- });
- ```
Options
Name | Type | Default | Description |
---|---|---|---|
--- | --- | --- | --- |
strings | String | null | Strings |
cursor | String | Pipe | String |
delay | 'natural' | 'natural' | The |
deleteSpeed | 'natural' | 'natural' | The |
loop | Boolean | false | Whether |
autoStart | Boolean | false | Whether |
pauseFor | Number | 1500 | The |
devMode | Boolean | false | Whether |
skipAddStyles | Boolean | Skip | |
wrapperClassName | String | 'Typewriter__wrapper' | Class |
cursorClassName | String | 'Typewriter__cursor' | Class |
stringSplitter | Function | String | |
onCreateTextNode | Function | null | Callback |
onRemoveNode | Function | null | Callback |
Methods
All methods can be chained together.
Name | Params | Description |
---|---|---|
--- | --- | --- |
start | - | Start |
stop | - | Stop |
pauseFor | ``ms`` | Pause |
typeString | ``string`` | Type |
pasteString | ``string`` | Paste |
deleteAll | ``speed`` | Delete |
deleteChars | ``amount`` | Delete |
callFunction | ``cb`` | Call |
changeDeleteSpeed | ``speed`` | The |
changeDelay | ``delay`` | Change |
Examples
Basic example
- ```js
- var app = document.getElementById('app');
- var typewriter = new Typewriter(app, {
- loop: true,
- delay: 75,
- });
- typewriter
- .pauseFor(2500)
- .typeString('A simple yet powerful native javascript')
- .pauseFor(300)
- .deleteChars(10)
- .typeString('<strong>JS</strong> plugin for a cool typewriter effect and ')
- .typeString('<strong>only <span style="color: #27ae60;">5kb</span> Gzipped!</strong>')
- .pauseFor(1000)
- .start();
- ```
Custom text node creator using callback
- ```js
- var app = document.getElementById('app');
- var customNodeCreator = function(character) {
- return document.createTextNode(character);
- }
- var typewriter = new Typewriter(app, {
- loop: true,
- delay: 75,
- onCreateTextNode: customNodeCreator,
- });
- typewriter
- .typeString('A simple yet powerful native javascript')
- .pauseFor(300)
- .start();
- ```
Custom handling text insert using input placeholder
- ```js
- var input = document.getElementById('input')
- var customNodeCreator = function(character) {
- // Add character to input placeholder
- input.placeholder = input.placeholder + character;
- // Return null to skip internal adding of dom node
- return null;
- }
- var onRemoveNode = function({ character }) {
- if(input.placeholder) {
- // Remove last character from input placeholder
- input.placeholder = input.placeholder.slice(0, -1)
- }
- }
- var typewriter = new Typewriter(null, {
- loop: true,
- delay: 75,
- onCreateTextNode: customNodeCreator,
- onRemoveNode: onRemoveNode,
- });
- typewriter
- .typeString('A simple yet powerful native javascript')
- .pauseFor(300)
- .start();
- ```
React
This includes a React component which can be used within your project. You can pass in a onInit function which will be called with the instance of the typewriter so you can use the typewriter core API.
- ```jsx
- import Typewriter from 'typewriter-effect';
- <Typewriter
- onInit={(typewriter) => {
- typewriter.typeString('Hello World!')
- .callFunction(() => {
- console.log('String typed out!');
- })
- .pauseFor(2500)
- .deleteAll()
- .callFunction(() => {
- console.log('All strings were deleted');
- })
- .start();
- }}
- />
- ```
Alternatively you can also pass in options to use auto play and looping for example:
- ```jsx
- import Typewriter from 'typewriter-effect';
- <Typewriter
- options={{
- strings: ['Hello', 'World'],
- autoStart: true,
- loop: true,
- }}
- />
- ```