README
SVG Optimizer is a Node.js-based tool for optimizing SVG vector graphics files.
Why?
SVG files, especially those exported from various editors, usually contain a lot of redundant and useless information. This can include editor metadata, comments, hidden elements, default or non-optimal values and other stuff that can be safely removed or converted without affecting the SVG rendering result.
Installation
- ```sh
- # Via npm
- npm -g install svgo
- # Via yarn
- yarn global add svgo
- ```
CLI usage
- ```sh
- # Processing single files:
- svgo one.svg two.svg -o one.min.svg two.min.svg
- # Processing directory of svg files, recursively using `-f`, `--folder` :
- svgo -f ./path/to/folder/with/svg/files -o ./path/to/folder/with/svg/output
- # Help for advanced usage
- svgo --help
- ```
Configuration
SVGO has a plugin-based architecture, separate plugins allows various xml svg optimizations. See built-in plugins.
SVGO automatically loads configuration from svgo.config.js or from --config ./path/myconfig.js. Some general options can be configured via CLI.
- ``` js
- // svgo.config.js
- module.exports = {
- multipass: true, // boolean. false by default
- datauri: 'enc', // 'base64' (default), 'enc' or 'unenc'.
- js2svg: {
- indent: 2, // string with spaces or number of spaces. 4 by default
- pretty: true, // boolean, false by default
- },
- plugins: [
- // set of built-in plugins enabled by default
- 'preset-default',
- // enable built-in plugins by name
- 'prefixIds',
- // or by expanded notation which allows to configure plugin
- {
- name: 'sortAttrs',
- params: {
- xmlnsOrder: 'alphabetical',
- },
- },
- ],
- };
- ```
Default preset
When extending default configuration specify preset-default plugin to enable optimisations.
Each plugin of default preset can be disabled or configured with "overrides" param.
- ``` js
- module.exports = {
- plugins: [
- {
- name: 'preset-default',
- params: {
- overrides: {
- // customize default plugin options
- inlineStyles: {
- onlyMatchedOnce: false,
- },
- // or disable plugins
- removeDoctype: false,
- },
- },
- },
- ],
- };
- ```
Default preset includes the following list of plugins:
- removeDoctype
- removeXMLProcInst
- removeComments
- removeMetadata
- removeEditorsNSData
- cleanupAttrs
- mergeStyles
- inlineStyles
- minifyStyles
- cleanupIds
- removeUselessDefs
- cleanupNumericValues
- convertColors
- removeUnknownsAndDefaults
- removeNonInheritableGroupAttrs
- removeUselessStrokeAndFill
- removeViewBox
- cleanupEnableBackground
- removeHiddenElems
- removeEmptyText
- convertShapeToPath
- convertEllipseToCircle
- moveElemsAttrsToGroup
- moveGroupAttrsToElems
- collapseGroups
- convertPathData
- convertTransform
- removeEmptyAttrs
- removeEmptyContainers
- mergePaths
- removeUnusedNS
- sortDefsChildren
- removeTitle
- removeDesc
Custom plugin
It's also possible to specify a custom plugin:
- ``` js
- const anotherCustomPlugin = require('./another-custom-plugin.js');
- module.exports = {
- plugins: [
- {
- name: 'customPluginName',
- params: {
- optionName: 'optionValue',
- },
- fn: (ast, params, info) => {},
- },
- anotherCustomPlugin,
- ],
- };
- ```
API usage
SVGO provides a few low level utilities.
optimize
The core of SVGO is optimize function.
- ``` js
- const { optimize } = require('svgo');
- const result = optimize(svgString, {
- // optional but recommended field
- path: 'path-to.svg',
- // all config fields are also available here
- multipass: true,
- });
- const optimizedSvgString = result.data;
- ```
loadConfig
If you write a tool on top of SVGO you might need a way to load SVGO config.
- ``` js
- const { loadConfig } = require('svgo');
- const config = await loadConfig();
- // you can also specify a relative or absolute path and customize the current working directory
- const config = await loadConfig(configFile, cwd);
- ```
Built-in plugins
Plugin | Description | Default |
---|---|---|
------------------------------------------------------------------------------------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------- | ---------- |
[cleanupAttrs](https://github.com/svg/svgo/blob/main/plugins/cleanupAttrs.js) | cleanup | `enabled` |
[mergeStyles](https://github.com/svg/svgo/blob/main/plugins/mergeStyles.js) | merge | `enabled` |
[inlineStyles](https://github.com/svg/svgo/blob/main/plugins/inlineStyles.js) | move | `enabled` |
[removeDoctype](https://github.com/svg/svgo/blob/main/plugins/removeDoctype.js) | remove | `enabled` |
[removeXMLProcInst](https://github.com/svg/svgo/blob/main/plugins/removeXMLProcInst.js) | remove | `enabled` |
[removeComments](https://github.com/svg/svgo/blob/main/plugins/removeComments.js) | remove | `enabled` |
[removeMetadata](https://github.com/svg/svgo/blob/main/plugins/removeMetadata.js) | remove | `enabled` |
[removeTitle](https://github.com/svg/svgo/blob/main/plugins/removeTitle.js) | remove | `enabled` |
[removeDesc](https://github.com/svg/svgo/blob/main/plugins/removeDesc.js) | remove | `enabled` |
[removeUselessDefs](https://github.com/svg/svgo/blob/main/plugins/removeUselessDefs.js) | remove | `enabled` |
[removeXMLNS](https://github.com/svg/svgo/blob/main/plugins/removeXMLNS.js) | removes | `disabled` |
[removeEditorsNSData](https://github.com/svg/svgo/blob/main/plugins/removeEditorsNSData.js) | remove | `enabled` |
[removeEmptyAttrs](https://github.com/svg/svgo/blob/main/plugins/removeEmptyAttrs.js) | remove | `enabled` |
[removeHiddenElems](https://github.com/svg/svgo/blob/main/plugins/removeHiddenElems.js) | remove | `enabled` |
[removeEmptyText](https://github.com/svg/svgo/blob/main/plugins/removeEmptyText.js) | remove | `enabled` |
[removeEmptyContainers](https://github.com/svg/svgo/blob/main/plugins/removeEmptyContainers.js) | remove | `enabled` |
[removeViewBox](https://github.com/svg/svgo/blob/main/plugins/removeViewBox.js) | remove | `enabled` |
[cleanupEnableBackground](https://github.com/svg/svgo/blob/main/plugins/cleanupEnableBackground.js) | remove | `enabled` |
[minifyStyles](https://github.com/svg/svgo/blob/main/plugins/minifyStyles.js) | minify | `enabled` |
[convertStyleToAttrs](https://github.com/svg/svgo/blob/main/plugins/convertStyleToAttrs.js) | convert | `disabled` |
[convertColors](https://github.com/svg/svgo/blob/main/plugins/convertColors.js) | convert | `enabled` |
[convertPathData](https://github.com/svg/svgo/blob/main/plugins/convertPathData.js) | convert | `enabled` |
[convertTransform](https://github.com/svg/svgo/blob/main/plugins/convertTransform.js) | collapse | `enabled` |
[removeUnknownsAndDefaults](https://github.com/svg/svgo/blob/main/plugins/removeUnknownsAndDefaults.js) | remove | `enabled` |
[removeNonInheritableGroupAttrs](https://github.com/svg/svgo/blob/main/plugins/removeNonInheritableGroupAttrs.js) | remove | `enabled` |
[removeUselessStrokeAndFill](https://github.com/svg/svgo/blob/main/plugins/removeUselessStrokeAndFill.js) | remove | `enabled` |
[removeUnusedNS](https://github.com/svg/svgo/blob/main/plugins/removeUnusedNS.js) | remove | `enabled` |
[prefixIds](https://github.com/svg/svgo/blob/main/plugins/prefixIds.js) | prefix | `disabled` |
[cleanupIds](https://github.com/svg/svgo/blob/main/plugins/cleanupIds.js) | remove | `enabled` |
[cleanupNumericValues](https://github.com/svg/svgo/blob/main/plugins/cleanupNumericValues.js) | round | `enabled` |
[cleanupListOfValues](https://github.com/svg/svgo/blob/main/plugins/cleanupListOfValues.js) | round | `disabled` |
[moveElemsAttrsToGroup](https://github.com/svg/svgo/blob/main/plugins/moveElemsAttrsToGroup.js) | move | `enabled` |
[moveGroupAttrsToElems](https://github.com/svg/svgo/blob/main/plugins/moveGroupAttrsToElems.js) | move | `enabled` |
[collapseGroups](https://github.com/svg/svgo/blob/main/plugins/collapseGroups.js) | collapse | `enabled` |
[removeRasterImages](https://github.com/svg/svgo/blob/main/plugins/removeRasterImages.js) | remove | `disabled` |
[mergePaths](https://github.com/svg/svgo/blob/main/plugins/mergePaths.js) | merge | `enabled` |
[convertShapeToPath](https://github.com/svg/svgo/blob/main/plugins/convertShapeToPath.js) | convert | `enabled` |
[convertEllipseToCircle](https://github.com/svg/svgo/blob/main/plugins/convertEllipseToCircle.js) | convert | `enabled` |
[sortAttrs](https://github.com/svg/svgo/blob/main/plugins/sortAttrs.js) | sort | `enabled` |
[sortDefsChildren](https://github.com/svg/svgo/blob/main/plugins/sortDefsChildren.js) | sort | `enabled` |
[removeDimensions](https://github.com/svg/svgo/blob/main/plugins/removeDimensions.js) | remove | `disabled` |
[removeAttrs](https://github.com/svg/svgo/blob/main/plugins/removeAttrs.js) | remove | `disabled` |
[removeAttributesBySelector](https://github.com/svg/svgo/blob/main/plugins/removeAttributesBySelector.js) | removes | `disabled` |
[removeElementsByAttr](https://github.com/svg/svgo/blob/main/plugins/removeElementsByAttr.js) | remove | `disabled` |
[addClassesToSVGElement](https://github.com/svg/svgo/blob/main/plugins/addClassesToSVGElement.js) | add | `disabled` |
[addAttributesToSVGElement](https://github.com/svg/svgo/blob/main/plugins/addAttributesToSVGElement.js) | adds | `disabled` |
[removeOffCanvasPaths](https://github.com/svg/svgo/blob/main/plugins/removeOffCanvasPaths.js) | removes | `disabled` |
[removeStyleElement](https://github.com/svg/svgo/blob/main/plugins/removeStyleElement.js) | remove | `disabled` |
[removeScriptElement](https://github.com/svg/svgo/blob/main/plugins/removeScriptElement.js) | remove | `disabled` |
[reusePaths](https://github.com/svg/svgo/blob/main/plugins/reusePaths.js) | Find | `disabled` |
Other Ways to Use SVGO
- as a web app – SVGOMG
- as a GitHub Action – SVGO Action
- as a Grunt task – grunt-svgmin
- as a Gulp task – gulp-svgmin
- as a Mimosa module – mimosa-minify-svg
- as an OSX Folder Action – svgo-osx-folder-action
- as a webpack loader – image-webpack-loader
- as a Telegram Bot – svgo_bot
- as a PostCSS plugin – postcss-svgo
- as an Inkscape plugin – inkscape-svgo
- as a Sketch plugin - svgo-compressor
- as a macOS app - Image Shrinker
- as a Rollup plugin - rollup-plugin-svgo
- as a VS Code plugin - vscode-svgo
- as a Atom plugin - atom-svgo
- as a Sublime plugin - Sublime-svgo
- as a Figma plugin - Advanced SVG Export
- as a Linux app - Oh My SVG
- as a Browser extension - SVG Gobbler
- as an API - Vector Express
Donators
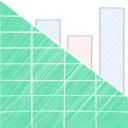
| :------------------------------------------------------------------------------: | :---------------------------------------------------------------------------------------------------------------------------: |
| SheetJS LLC | Fontello |
License and Copyright
This software is released under the terms of the MIT license.
Logo by André Castillo.