Suspensive
Packages to use React Suspense easily
README
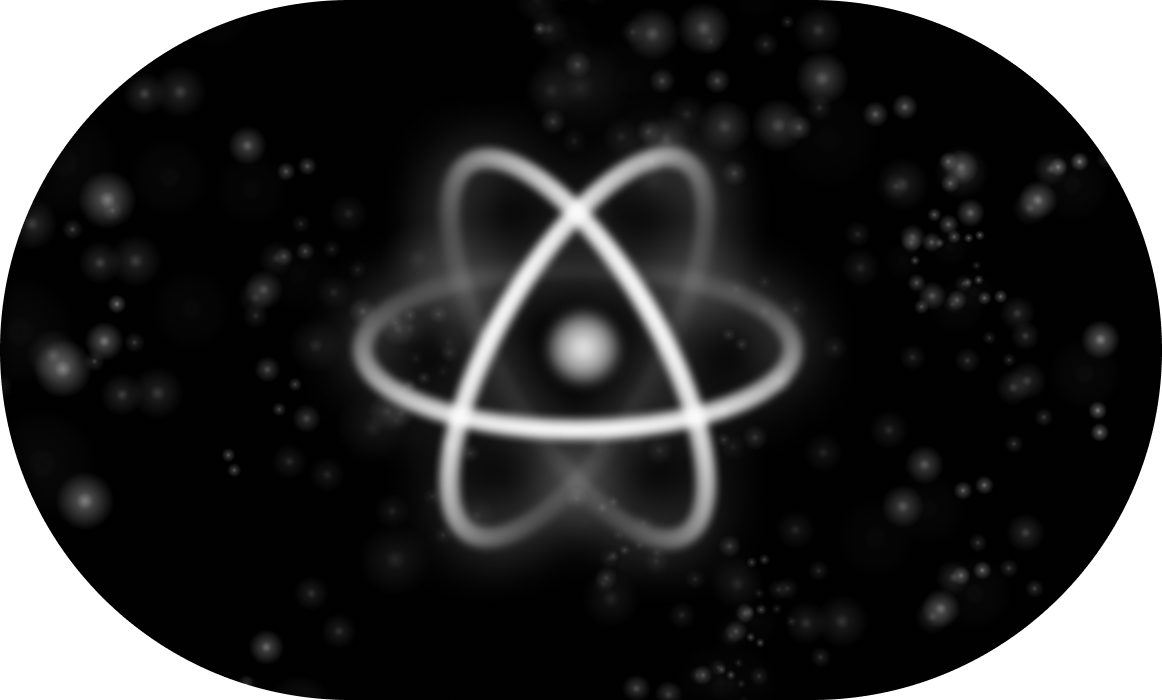
All declarative components to use suspense on both CSR, SSR.
Features
- Suspense, withSuspense (containing CSROnly)
- ErrorBoundary, withErrorBoundary, useErrorBoundary, useErrorBoundaryFallbackProps
- ErrorBoundaryGroup, withErrorBoundaryGroup, useErrorBoundaryGroup
- AsyncBoundary, withAsyncBoundary (containing CSROnly)
- Delay, withDelay
- SuspensiveProvider, Suspensive
Installation
- ```shell
- npm install @suspensive/react
- ```
- ```shell
- pnpm add @suspensive/react
- ```
- ```shell
- yarn add @suspensive/react
- ```
Usage
- ```tsx
- import { Suspense, ErrorBoundary, ErrorBoundaryGroup, Delay } from '@suspensive/react'
- const Example = () => (
- <ErrorBoundaryGroup>
- <ErrorBoundaryGroup.Reset trigger={(group) => <button onClick={group.reset}>Reset All</button>} />
- <ErrorBoundary
- fallback={(props) => (
- <>
- <button onClick={props.reset}>Try again</button>
- {props.error.message}
- </>
- )}
- >
- <Suspense
- fallback={
- <Delay>
- <Spinner />
- </Delay>
- }
- >
- <SuspendedComponent />
- </Suspense>
- </ErrorBoundary>
- </ErrorBoundaryGroup>
- )
- ```
Declarative apis to use @tanstack/react-query with suspense easily.
Features
- useSuspenseQuery
- useSuspenseQueries
- useSuspenseInfiniteQuery
- QueryErrorBoundary, QueryAsyncBoundary
Installation
- ```shell
- npm install @suspensive/react-query
- ```
- ```shell
- pnpm add @suspensive/react-query
- ```
- ```shell
- yarn add @suspensive/react-query
- ```
Usage
- ```tsx
- import { Suspense } from '@suspensive/react'
- import { QueryErrorBoundary, useSuspenseQuery } from '@suspensive/react-query'
- const Example = () => (
- <QueryErrorBoundary
- fallback={(props) => (
- <>
- <button onClick={props.reset}>Try again</button>
- {props.error.message}
- </>
- )}
- >
- <Suspense fallback={<Spinner />}>
- <SuspendedComponent />
- </Suspense>
- </QueryErrorBoundary>
- )
- const SuspendedComponent = () => {
- const query = useSuspenseQuery({
- queryKey,
- queryFn,
- })
- return <>{query.data}</>
- }
- ```
Docs
We provide Official Docs
See OFFICIAL DOCS
Visualization
Concepts Visualization ready. You can see core concepts of Suspensive visually
See VISUALIZATION.
Contributing
Read our Contributing Guide to familiarize yourself with Suspensive's development process, how to suggest bug fixes and improvements, and the steps for building and testing your changes.
Contributors
License
MIT © Suspensive. See LICENSE for details.