React Trello
Pluggable components to add a kanban board to your application
README
React Trello
Pluggable components to add a Trello (like) kanban board to your application
This library is not affiliated, associated, authorized, endorsed by or in any way officially connected to Trello, Inc. Trello is a registered trademark of Atlassian, Inc.
Features Showcase
Features
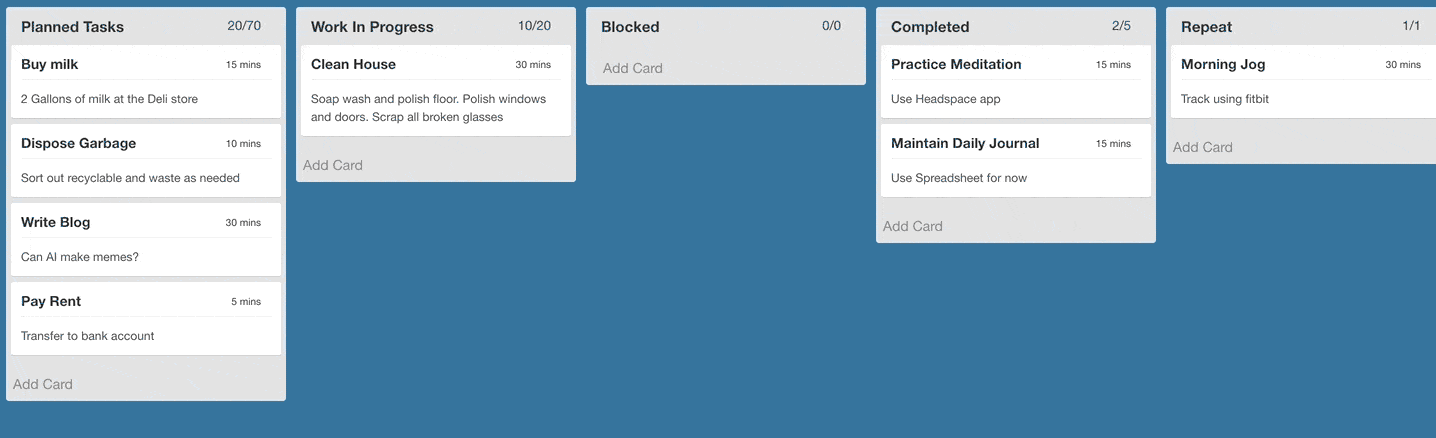
Responsive and extensible
Easily pluggable into existing react application
Supports pagination when scrolling individual lanes
Drag-And-Drop on cards and lanes (compatible with touch devices)
Edit functionality to add/delete cards
Custom elements to define lane and card appearance
Event bus for triggering events externally (e.g.: adding or removing cards based on events coming from backend)
Inline edit lane's title
Getting Started
Install using npm or yarn
- ``` sh
- $ npm install --save react-trello
- ```
or
- ``` sh
- $ yarn add react-trello
- ```
Usage
The Board component takes a prop called data that contains all the details related to rendering the board. A sample data json is given here to illustrate the contract:
- ``` js
- const data = {
- lanes: [
- {
- id: 'lane1',
- title: 'Planned Tasks',
- label: '2/2',
- cards: [
- {id: 'Card1', title: 'Write Blog', description: 'Can AI make memes', label: '30 mins', draggable: false},
- {id: 'Card2', title: 'Pay Rent', description: 'Transfer via NEFT', label: '5 mins', metadata: {sha: 'be312a1'}}
- ]
- },
- {
- id: 'lane2',
- title: 'Completed',
- label: '0/0',
- cards: []
- }
- ]
- }
- ```
draggable property of Card object is true by default.
The data is passed to the board component and that's it.
- ``` js
- import React from 'react'
- import Board from 'react-trello'
- export default class App extends React.Component {
- render() {
- return <Board data={data} />
- }
- }
- ```
Refer to storybook for detailed examples: https://rcdexta.github.io/react-trello/
Also refer to the sample project that uses react-trello as illustration: https://github.com/rcdexta/react-trello-example
Use edge version of project (current master branch)
- ``` sh
- $ yarn add rcdexta/react-trello
- ```
and
- ``` js
- import Board from 'react-trello/src'
- ```
Upgrade
Breaking changes. Since version 2.2 these properties are removed: addLaneTitle, addCardLink, customLaneHeader, newCardTemplate, newLaneTemplate,
and customCardLayout with children element.
Follow upgrade instructions to make easy migration.
Properties
This is the container component that encapsulates the lanes and cards
Required parameters
Name | Type | Description |
---|---|---|
------------------- | -------- | ------------------------------------------------------------------------------------------------------------------------------ |
data | object | Actual |
Optionable flags
Name | Type | Description |
---|---|---|
------------------- | -------- | ------------------------------------------------------------------------------------------------------------------------------ |
draggable | boolean | Makes |
laneDraggable | boolean | Set |
cardDraggable | boolean | Set |
collapsibleLanes | boolean | Make |
editable | boolean | Makes |
canAddLanes | boolean | Allows |
hideCardDeleteIcon | boolean | Disable |
editLaneTitle | boolean | Allow |
Callbacks and handlers
Name | Type | Description |
---|---|---|
------------------- | -------- | ------------------------------------------------------------------------------------------------------------------------------ |
handleDragStart | function | Callback |
handleDragEnd | function | Callback |
handleLaneDragStart | function | Callback |
handleLaneDragEnd | function | Callback |
onDataChange | function | Called |
onCardClick | function | Called |
onCardAdd | function | Called |
onBeforeCardDelete | function | Called |
onCardDelete | function | Called |
onCardMoveAcrossLanes | function | Called |
onLaneAdd | function | Called |
onLaneDelete | function | Called |
onLaneUpdate | function | Called |
onLaneClick | function | Called |
onLaneScroll | function | Called |
Other functions
Name | Type | Description |
---|---|---|
------------------- | -------- | ------------------------------------------------------------------------------------------------------------------------------ |
eventBusHandle | function | This |
laneSortFunction | function | Used |
I18n support
Name | Type | Description |
---|---|---|
------------------- | -------- | ------------------------------------------------------------------------------------------------------------------------------ |
lang | string | Language |
t | function | Translation |
Style customization
Name | Type | Description |
---|---|---|
------------------- | -------- | ------------------------------------------------------------------------------------------------------------------------------ |
style | object | Pass |
cardStyle | object | CSS |
laneStyle | object | CSS |
tagStyle | object | If |
cardDragClass | string | CSS |
cardDropClass | string | CSS |
laneDragClass | string | CSS |
laneDropClass | string | CSS |
components | object | Map |
Lane specific props
Name | Type | Description |
---|---|---|
------------------- | -------- | ------------------------------------------------------------------------------------------------------------------------------ |
id | string | ID |
style | object | Pass |
labelStyle | object | Pass |
cardStyle | object | Pass |
disallowAddingCard | boolean | Disallow |
Refer to stories folder for examples on many more options for customization.
Editable Board
It is possible to make the entire board editable by setting the editable prop to true. This switch prop will enable existing cards to be deleted and show a Add Card link at the bottom of each lane, clicking which will show an inline editable new card.
Check out the editable board story and its corresponding source code for more details.
Styling and customization
There are three ways to apply styles to the library components including Board, Lane or Card:
1. Predefined CSS classnames
Use the predefined css classnames attached to these elements that go by .react-trello-lane, .react-trello-card, .react-trello-board:
- ```css
- .react-trello-lane {
- border: 0;
- background-color: initial;
- }
- ```
2. Pass custom style attributes as part of data.
This method depends on used Card and Lane components.
- ``` js
- const data = {
- lanes: [
- {
- id: 'lane1',
- title: 'Planned Tasks',
- style: { backgroundColor: 'yellow' }, // Style of Lane
- cardStyle: { backgroundColor: 'blue' } // Style of Card
- ...
- };
- <Board
- style={{backgroundColor: 'red'}} // Style of BoardWrapper
- data={data}
- />
- ```
Storybook example - stories/Styling.story.js
3. Completely customize the look-and-feel by using components dependency injection.
You can override any of used components (ether one or completery all)
- ``` js
- const components = {
- GlobalStyle: MyGlobalStyle, // global style created with method `createGlobalStyle` of `styled-components`
- LaneHeader: MyLaneHeader,
- Card: MyCard,
- AddCardLink: MyAddCardLink,
- ...
- };
- <Board components={components} />
- ```
Total list of customizable components: src/components/index.js
Refer to components definitions to discover their properties list and types.
Refer more examples in storybook.
Publish Events
When defining the board, it is possible to obtain a event hook to the component to publish new events later after the board has been rendered. Refer the example below:
- ``` js
- let eventBus = undefined
- const setEventBus = (handle) => {
- eventBus = handle
- }
- //To add a card
- eventBus.publish({type: 'ADD_CARD', laneId: 'COMPLETED', card: {id: "M1", title: "Buy Milk", label: "15 mins", description: "Also set reminder"}})
- //To update a card
- eventBus.publish({type: 'UPDATE_CARD', laneId: 'COMPLETED', card: {id: "M1", title: "Buy Milk (Updated)", label: "20 mins", description: "Also set reminder (Updated)"}})
- //To remove a card
- eventBus.publish({type: 'REMOVE_CARD', laneId: 'PLANNED', cardId: "M1"})
- //To move a card from one lane to another. index specifies the position to move the card to in the target lane
- eventBus.publish({type: 'MOVE_CARD', fromLaneId: 'PLANNED', toLaneId: 'WIP', cardId: 'Plan3', index: 0})
- //To update the lanes
- eventBus.publish({type: 'UPDATE_LANES', lanes: newLaneData})
- <Board data={data} eventBusHandle={setEventBus}/>
- ```
The first event in the above example will move the card Buy Milk from the planned lane to completed lane. We expect that this library can be wired to a backend push api that can alter the state of the board in realtime.
I18n and text translations
Custom text translation function
Pass translation function to provide custom or localized texts:
- ``` js
- // If your translation table is flat
- //
- // For example: { 'placeholder.title': 'some text' }
- const customTranslation = (key) => TRANSLATION_TABLE[key]
- // If your translation table has nested hashes (provided translations table is it)
- //
- // For example: { 'placeholder': { 'title': 'some text' } }
- import { createTranslate } from 'react-trello'
- const customTranslation = createTranslate(TRANSLATION_TABLE)
- <Board t={customTranslation} .../>
- ```
List of available keys - locales/en/translation.json
react-i18next example
- ``` js
- import { withTranslation } from 'react-i18next';
- const I18nBoard = withTranslation()(Board)
- ```
Compatible Browsers
Tested to work with following browsers using Browserling:
Chrome 60 or above
Firefox 52 or above
Opera 51 or above
Safari 4.0 or above
Microsoft Edge 15 or above
Logging
Pass environment variable REDUX_LOGGING as true to enable Redux logging in any environment
Development
- ```
- cd react-trello/
- yarn install
- yarn run storybook
- ```
Scripts
1. yarn run lint : Lint all js files
2. yarn run lintfix : fix linting errors of all js files
3. yarn run semantic-release : make a release. Leave it for CI to do.
4. yarn run storybook: Start developing by using storybook
5. yarn run test : Run tests. tests file should be written as *.test.js and using ES2015
6. yarn run test:watch : Watch tests while writing
7. yarn run test:cover : Show coverage report of your tests
8. yarn run test:report : Report test coverage to codecov.io. Leave this for CI
9. yarn run build: transpile all ES6 component files into ES5(commonjs) and put it in dist directory
10. yarn run docs: create static build of storybook in docs directory that can be used for github pages
Learn how to write stories here
Maintainers
rcdexta | dapi |
License
MIT