Postgres.js
The fastest full featured PostgreSQL client for Node.js, Deno, Bun and Clou...
README
Getting started
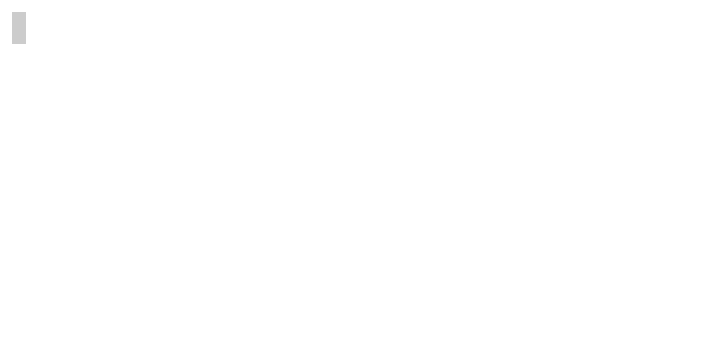
Installation
- ```bash
- $ npm install postgres
- ```
Usage
- ```js
- // db.js
- import postgres from 'postgres'
- const sql = postgres({ /* options */ }) // will use psql environment variables
- export default sql
- ```
- ```js
- // users.js
- import sql from './db.js'
- async function getUsersOver(age) {
- const users = await sql`
- select
- name,
- age
- from users
- where age > ${ age }
- `
- // users = Result [{ name: "Walter", age: 80 }, { name: 'Murray', age: 68 }, ...]
- return users
- }
- async function insertUser({ name, age }) {
- const users = await sql`
- insert into users
- (name, age)
- values
- (${ name }, ${ age })
- returning name, age
- `
- // users = Result [{ name: "Murray", age: 68 }]
- return users
- }
- ```
ESM dynamic imports
- ```js
- const { default: postgres } = await import('postgres')
- ```
Table of Contents
Connection
postgres([url], [options])
- ```js
- const sql = postgres('postgres://username:password@host:port/database', {
- host : '', // Postgres ip address[s] or domain name[s]
- port : 5432, // Postgres server port[s]
- database : '', // Name of database to connect to
- username : '', // Username of database user
- password : '', // Password of database user
- ...and more
- })
- ```
Queries
await sql... -> Result[]
- ```js
- const xs = await sql`
- insert into users (
- name, age
- ) values (
- 'Murray', 68
- )
- returning *
- `
- // xs = [{ user_id: 1, name: 'Murray', age: 68 }]
- ```
Please note that queries are first executed when awaited – or instantly by using [.execute()](#execute).
Query parameters
- ```js
- const name = 'Mur'
- , age = 60
- const users = await sql`
- select
- name,
- age
- from users
- where
- name like ${ name + '%' }
- and age > ${ age }
- `
- // users = [{ name: 'Murray', age: 68 }]
- ```
Be careful with quotation marks here. Because Postgres infers column types, you do not need to wrap your interpolated parameters in quotes like '${name}'. This will cause an error because the tagged template replaces ${name} with $1 in the query string, leaving Postgres to do the interpolation. If you wrap that in a string, Postgres will see '$1' and interpret it as a string as opposed to a parameter.
Dynamic column selection
- ```js
- const columns = ['name', 'age']
- await sql`
- select
- ${ sql(columns) }
- from users
- `
- // Which results in:
- select "name", "age" from users
- ```
Dynamic inserts
- ```js
- const user = {
- name: 'Murray',
- age: 68
- }
- await sql`
- insert into users ${
- sql(user, 'name', 'age')
- }
- `
- // Which results in:
- insert into users ("name", "age") values ($1, $2)
- // The columns can also be given with an array
- const columns = ['name', 'age']
- await sql`
- insert into users ${
- sql(user, columns)
- }
- `
- ```
Multiple inserts in one query
- ```js
- const users = [{
- name: 'Murray',
- age: 68,
- garbage: 'ignore'
- },
- {
- name: 'Walter',
- age: 80
- }]
- await sql`insert into users ${ sql(users, 'name', 'age') }`
- // Is translated to:
- insert into users ("name", "age") values ($1, $2), ($3, $4)
- // Here you can also omit column names which will use object keys as columns
- await sql`insert into users ${ sql(users) }`
- // Which results in:
- insert into users ("name", "age") values ($1, $2), ($3, $4)
- ```
Dynamic columns in updates
- ```js
- const user = {
- id: 1,
- name: 'Murray',
- age: 68
- }
- await sql`
- update users set ${
- sql(user, 'name', 'age')
- }
- where user_id = ${ user.id }
- `
- // Which results in:
- update users set "name" = $1, "age" = $2 where user_id = $3
- // The columns can also be given with an array
- const columns = ['name', 'age']
- await sql`
- update users set ${
- sql(user, columns)
- }
- where user_id = ${ user.id }
- `
- ```