dotenv-flow
Loads environment variables from .env.[development|test|production][.local]...
README
dotenv-flow
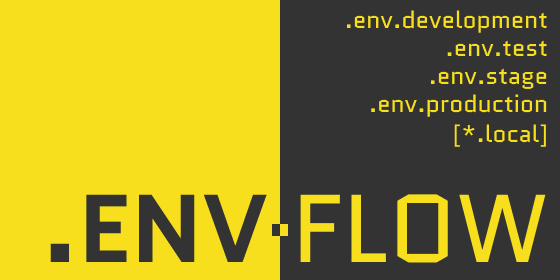
_dotenv is a zero-dependency npm module that loads environment variables from a.env file into [process.env](https://nodejs.org/docs/latest/api/process.html#process_process_env)._
dotenv-flow extends _dotenv_, adding support of NODE_ENV-specific `.env files _like .env.development, .env.test, .env.stage, and .env.production,_ and the appropriate .env.local` overrides.
It allows your app to have multiple environments _(like "development", "test", "stage", and "production" respectively)_ with selectively-adjusted environment variable setups and load them dynamically depending on the current NODE_ENV.
In addition to that, .env*.local overrides add the ability to overwrite variables locally for development, testing, and debugging purposes
_(note that the appropriate .env*.local entry should be added to your .gitignore)_.
🌱 Inspired by _Ruby's dotenv (a.k.a. dotenv-rails) gem_, _CreateReactApp's *storing configs in `.env` files approach_,
the _Twelve-Factor App methodology_ in general, and _its store config in the environment section_ in particular.
Installation
Using NPM:
- ```sh
- $ npm install dotenv-flow --save
- ```
Using Yarn:
- ```sh
- $ yarn add dotenv-flow
- ```
Using PNPM:
- ```sh
- $ pnpm add dotenv-flow
- ```
Usage
As early as possible in your Node.js app, initialize dotenv-flow:
- ```js
- require('dotenv-flow').config();
- ```
It will allow you to configure and use dotenv-flow from your code programmatically.
If you're using TypeScript or ES Modules:
- ```ts
- import dotenvFlow from 'dotenv-flow';
- dotenvFlow.config();
- ```
Alternatively, you can use the default config entry point that allows you to configure dotenv-flow using command switch flags or predefined environment variables:
- ```js
- require('dotenv-flow/config');
- ```
Or even make dotenv-flow load environment variables for your app without adding it to the code using preload technique:
- ```sh
- $ node -r "dotenv-flow/config" your_app.js
- ```
It works with ts-node as well:
- ```sh
- $ ts-node -r "dotenv-flow/config" your_app.ts
- ```
How it works
Once dotenv-flow is initialized (using .config or any other method above), environment variables defined in your `.env files are loaded and become accessible in your Node.js app via process.env.`.
For example, let's suppose that you have the following .env* files in your project:
- ```sh
- # .env
- DATABASE_HOST=127.0.0.1
- DATABASE_PORT=27017
- DATABASE_USER=default
- DATABASE_PASS=
- DATABASE_NAME=my_app
- ```
- ```sh
- # .env.local
- DATABASE_USER=local-user
- DATABASE_PASS=super-secret
- ```
- ```sh
- # .env.development
- DATABASE_NAME=my_app_dev
- ```
- ```sh
- # .env.test
- DATABASE_NAME=my_app_test
- ```
- ```sh
- # .env.production
- DATABASE_NAME=my_app_prod
- ```
- ```sh
- # .env.production.local
- DATABASE_HOST=10.0.0.32
- DATABASE_PORT=27017
- DATABASE_USER=devops
- DATABASE_PASS=1qa2ws3ed4rf5tg6yh
- DATABASE_NAME=application_storage
- ```
- ```js
- // your_script.js
- require('dotenv-flow').config();
- console.log('database host:', process.env.DATABASE_HOST);
- console.log('database port:', process.env.DATABASE_PORT);
- console.log('database user:', process.env.DATABASE_USER);
- console.log('database pass:', process.env.DATABASE_PASS);
- console.log('database name:', process.env.DATABASE_NAME);
- ```
And if you run your_script.js in the development environment, like:
- ```sh
- $ NODE_ENV=development node your_script.js
- ```
you'll get the following output:
- ```text
- database host: 127.0.0.1
- database port: 27017
- database user: local-user
- database pass: super-secret
- database name: my_app_dev
- ```
Or if you run the same script in the production environment:
- ```sh
- $ NODE_ENV=production node your_script.js
- ```
you'll get the following:
- ```text
- database host: 10.0.0.32
- database port: 27017
- database user: devops
- database pass: 1qa2ws3ed4rf5tg6yh
- database name: application_storage
- ```
Note that the .env*.local files should be ignored by your version control system (refer the Files under version control section below to learn more), and you should have the .env.production.local file only on your production deployment machine.