env-var
Verification, sanitization, and type coercion for environment variables in ...
README
env-var
Verification, sanitization, and type coercion for environment variables in Node.js and web applications. Supports TypeScript!
🏋 Lightweight. Zero dependencies and just ~4.7kB when minified!
🧹 Clean and simple code, as shown here.
🚫 Fails fast if your environment is misconfigured.
👩💻 Friendly error messages and example values for better debugging experience.
🎉 TypeScript support provides compile time safety and better developer experience.
📦 Support for frontend projects, e.g in React, React Native, Angular, etc.
Contents
- API: The full API set forenv-var
- Examples: Example usage ofenv-var
Install
npm
- ``` sh
- npm install env-var
- ```
yarn
- ``` sh
- yarn add env-var
- ```
Getting started
You can use env-var in both JavaScript and TypeScript!
Javascript example
- ``` js
- const env = require('env-var');
- // Or using module import syntax:
- // import env from 'env-var'
- const PASSWORD = env.get('DB_PASSWORD')
- // Throws an error if the DB_PASSWORD variable is not set (optional)
- .required()
- // Decode DB_PASSWORD from base64 to a utf8 string (optional)
- .convertFromBase64()
- // Call asString (or other APIs) to get the variable value (required)
- .asString();
- // Read in a port (checks that PORT is in the range 0 to 65535)
- // Alternatively, use a default value of 5432 if PORT is not defined
- const PORT = env.get('PORT').default('5432').asPortNumber()
- ```
TypeScript example
- ```ts
- import * as env from 'env-var';
- // Read a PORT environment variable and ensure it's a positive integer.
- // An EnvVarError will be thrown if the variable is not set, or if it
- // is not a positive integer.
- const PORT: number = env.get('PORT').required().asIntPositive();
- ```
For more examples, refer to the /example directory and EXAMPLE.md. A summary of the examples available in/example is written in the 'Other examples' section of EXAMPLE.md.
API
The examples above only cover a very small set of env-var API calls. There are many others such as asFloatPositive(), asJson() and asRegExp(). For a full list of env-var API calls, check out API.md.
You can also create your own custom accessor; refer to the 'extraAccessors' section of API.md.
Logging
Logging is disabled by default in env-var to prevent accidental logging of secrets.
To enable logging, you need to create an env-var instance using the from() function that the API provides and pass in a logger.
- A built-in logger is available, but a custom logger is also supported.
- Always exercise caution when logging environment variables!
Using the Built-in Logger
The built-in logger will print logs only when NODE_ENV is not set to either prod or production.
- ``` js
- const { from, logger } = require('env-var')
- const env = from(process.env, {}, logger)
- const API_KEY = env.get('API_KEY').required().asString()
- ```
This is an example output from the built-in logger generated by running example/logging.js:
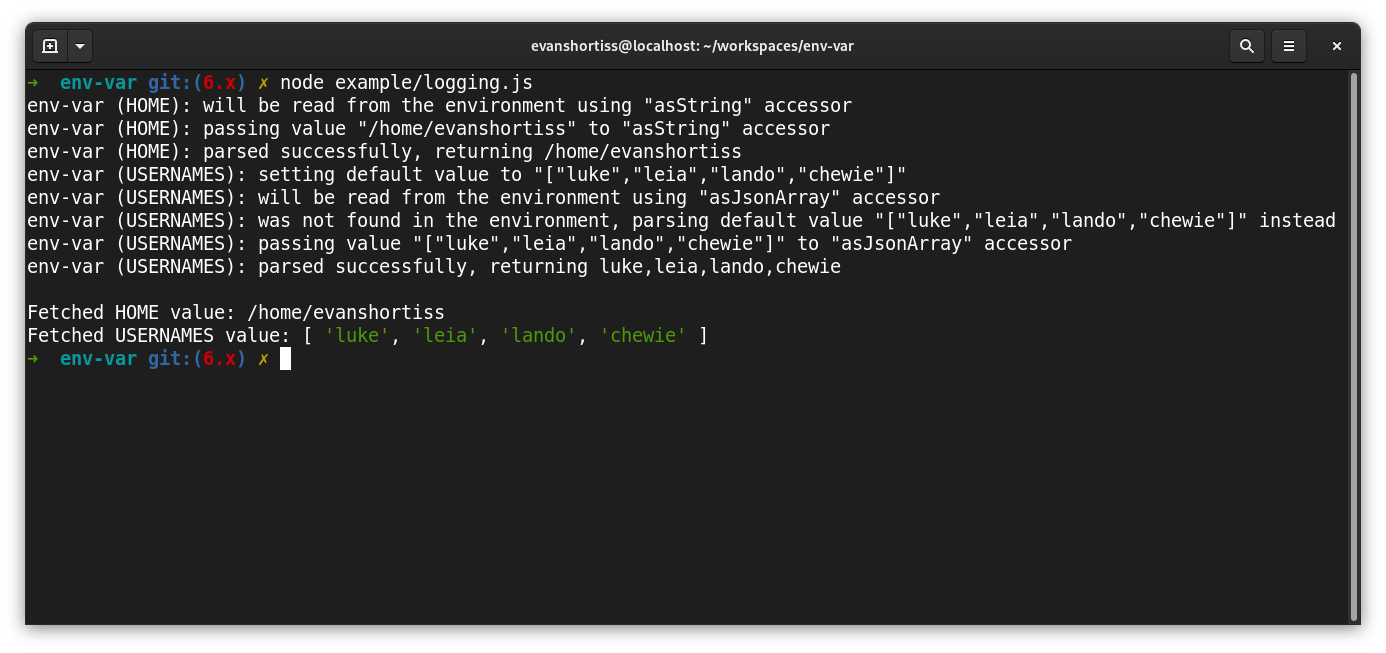
Using a Custom Logger
If you need to filter env-var logs based on log levels (e.g. trace logging only) or have your own preferred logger, you can use a custom logging solution such as pino easily.
See the 'Custom logging' section of EXAMPLE.md for more information.
Optional integration with dotenv
There is no coupling between dotenv and env-var, but you can easily use them both together. This loose coupling reduces package bloat and allows you to start or stop using one without being forced to do the same for the other.
See the 'dotenv' section of EXAMPLE.md for more information.
Contributing
Contributions are welcomed and discussed in CONTRIBUTING.md. If you would like to discuss an idea, open an issue or a PR with an initial implementation.
Contributors
@aautio
@avocadomaster
@caccialdo
@ChibiBlasphem
@DigiPie
@evanshortiss
@gabrieloczkowski
@hhravn
@ineentho
@itavy
@jerome-fox
@joh-klein
@Lioness100
@MikeyBurkman
@pepakriz
@rmblstrp
@shawnmclean
@todofixthis
@xuo