FlexLayout
A multi-tab layout manager
README
FlexLayout
FlexLayout is a layout manager that arranges React components in multiple tab sets, tabs can be resized and moved.
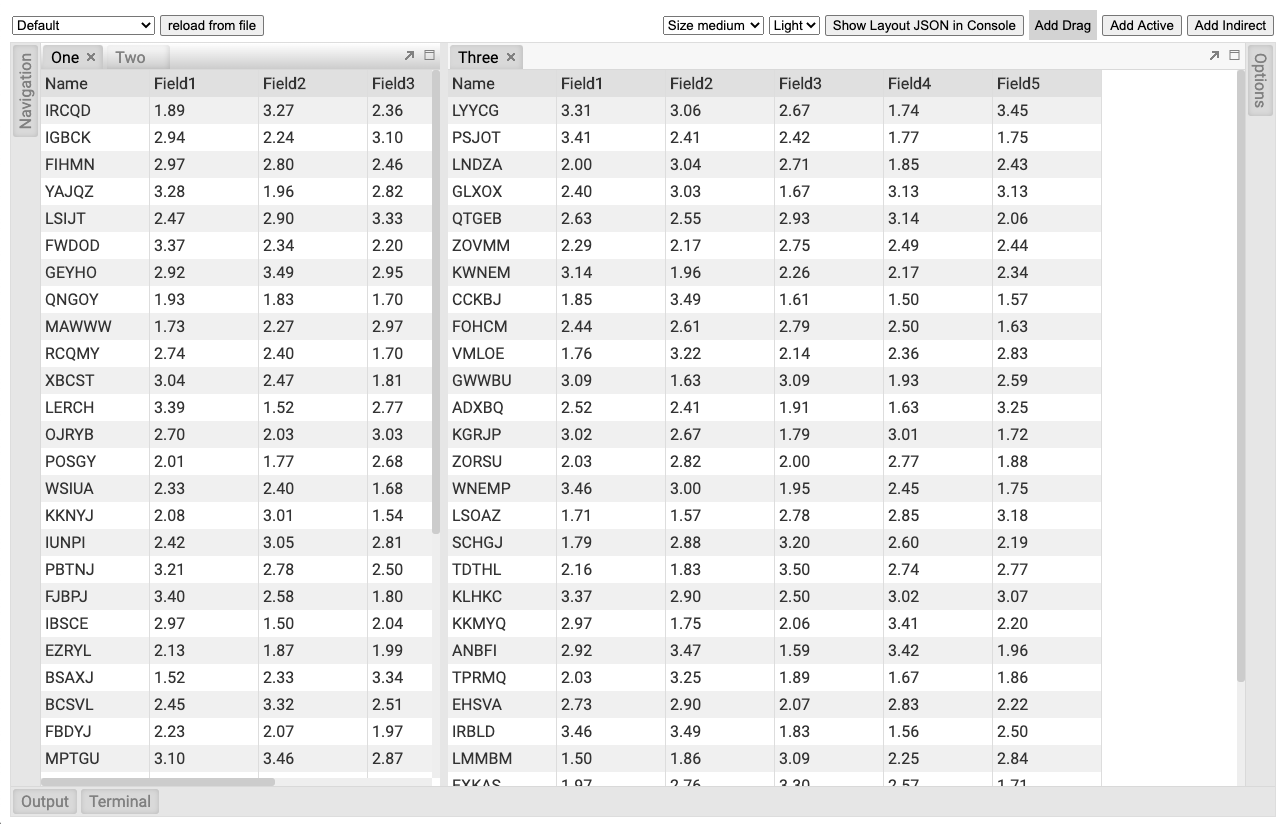
Try it now using JSFiddle
FlexLayout's only dependencies are React and uuid.
Features:
splitters
tabs
tab dragging and ordering
tabset dragging (move all the tabs in a tabset in one operation)
dock to tabset or edge of frame
maximize tabset (double click tabset header or use icon)
tab overflow (show menu when tabs overflow, scroll tabs using mouse wheel)
border tabsets
popout tabs into new browser windows
submodels, allow layouts inside layouts
tab renaming (double click tab text to rename)
theming - light, underline, gray and dark
touch events - works on mobile devices (iPad, Android)
add tabs using drag, indirect drag, add to active tabset, add to tabset by id
preferred pixel size tabsets (try to keep their size when window resizes)
headed tabsets
tab and tabset attributes: enableHeader, enableTabStrip, enableDock, enableDrop...
customizable tabs and tabset header rendering
typescript type declarations included
Installation
FlexLayout is in the npm repository. Simply install React and FlexLayout from npm:
- ```
- npm install react
- npm install react-dom
- npm install flexlayout-react
- ```
Import React and FlexLayout in your modules:
- ```
- import * as React from "react";
- import { createRoot } from "react-dom/client";
- import * as FlexLayout from "flexlayout-react";
- ```
Include the light, underline, gray or dark style in your html:
- ```
- <link rel="stylesheet" href="node_modules/flexlayout-react/style/light.css" />
- ```
Usage
Required props:
Prop | Description |
---|---|
--------------- | ----------------- |
model | the |
factory | a |
Additional optional props
The model is tree of Node objects that define the structure of the layout.
The factory is a function that takes a Node object and returns a React component that should be hosted by a tab in the layout.
The model can be created using the Model.fromJson(jsonObject) static method, and can be saved using the model.toJson() method.
- ``` js
- this.state = {model: FlexLayout.Model.fromJson(json)};
- render() {
- <FlexLayout.Layout model={this.state.model} factory={factory}/>
- }
- ```
Example Configuration:
- ``` js
- var json = {
- global: {},
- borders: [],
- layout: {
- type: "row",
- weight: 100,
- children: [
- {
- type: "tabset",
- weight: 50,
- children: [
- {
- type: "tab",
- name: "One",
- component: "button",
- }
- ]
- },
- {
- type: "tabset",
- weight: 50,
- children: [
- {
- type: "tab",
- name: "Two",
- component: "button",
- }
- ]
- }
- ]
- }
- };
- ```
Example Code
- ```
- import * as React from "react";
- import { createRoot } from "react-dom/client";
- import * as FlexLayout from "flexlayout-react";
- class Main extends React.Component {
- constructor(props) {
- super(props);
- this.state = {model: FlexLayout.Model.fromJson(json)};
- }
- factory = (node) => {
- var component = node.getComponent();
- if (component === "button") {
- return <button>{node.getName()}</button>;
- }
- }
- render() {
- return (
- <FlexLayout.Layout model={this.state.model} factory={this.factory}/>
- )
- }
- }
- const root = createRoot(document.getElementById("container"));
- root.render(<Main/>);
- ```
The above code would render two tabsets horizontally each containing a single tab that hosts a button component. The tabs could be moved and resized by dragging and dropping. Additional grids could be added to the layout by sending actions to the model.
Try it now using JSFiddle
A simple Typescript example can be found here:
https://github.com/nealus/FlexLayout_cra_example
The model is built up using 4 types of 'node':
row - rows contains a list of tabsets and child rows, the top level 'row' will render horizontally (unless the global attribute rootOrientationVertical is set)
, child 'rows' will render in the opposite orientation to their parent.
tabset - tabsets contain a list of tabs and the index of the selected tab
tab - tabs specify the name of the component that they should host (that will be loaded via the factory) and the text of the actual tab.
border - borders contain a list of tabs and the index of the selected tab, they can only be used in the borders
top level element.
The main layout is defined with rows within rows that contain tabsets that themselves contain tabs.
The model json contains 3 top level elements:
global - where global options are defined
layout - where the main row/tabset/tabs layout hierarchy is defined
borders - (optional) where up to 4 borders are defined ("top", "bottom", "left", "right").
Weights on rows and tabsets specify the relative weight of these nodes within the parent row, the actual values do not matter just their relative values (ie two tabsets of weights 30,70 would render the same if they had weights of 3,7).
NOTE: the easiest way to create your initial layout JSON is to use the demo app, modify one of the
existing layouts by dragging/dropping and adding nodes then press the 'Show Layout JSON in console' button to print the JSON to the browser developer console.
example borders section:
- ```
- borders: [
- {
- type: "border",
- location: "left",
- children: [
- {
- type: "tab",
- enableClose: false,
- name: "Navigation",
- component: "grid",
- }
- ]
- },
- {
- type: "border",
- location: "right",
- children: [
- {
- type: "tab",
- enableClose: false,
- name: "Options",
- component: "grid",
- }
- ]
- },
- {
- type: "border",
- location: "bottom",
- children: [
- {
- type: "tab",
- enableClose: false,
- name: "Activity Blotter",
- component: "grid",
- },
- {
- type: "tab",
- enableClose: false,
- name: "Execution Blotter",
- component: "grid",
- }
- ]
- }
- ]
- ```
To control where nodes can be dropped you can add a callback function to the model:
- ```
- model.setOnAllowDrop(this.allowDrop);
- ```
example:
- ```
- allowDrop(dragNode, dropInfo) {
- let dropNode = dropInfo.node;
- // prevent non-border tabs dropping into borders
- if (dropNode.getType() == "border" && (dragNode.getParent() == null || dragNode.getParent().getType() != "border"))
- return false;
- // prevent border tabs dropping into main layout
- if (dropNode.getType() != "border" && (dragNode.getParent() != null && dragNode.getParent().getType() == "border"))
- return false;
- return true;
- }
- ```
By changing global or node attributes you can change the layout appearance and functionality, for example:
Setting tabSetEnableTabStrip:false in the global options would change the layout into a multi-splitter (without
tabs or drag and drop).
- ```
- global: {tabSetEnableTabStrip:false},
- ```
Floating Tabs (Popouts)
Tabs can be rendered into external browser windows (for use in multi-monitor setups)
by configuring them with the enableFloat attribute. When this attribute is present
an additional icon is shown in the tab header bar allowing the tab to be popped out
into an external window.
For popouts to work there needs to be an additional html page 'popout.html' hosted
at the same location as the main page (copy the one from examples/demo). The popout.html is the host page for the
popped out tab, the styles from the main page will be copied into it at runtime.
Because popouts are rendering into a different document to the main layout any code in the popped out
tab that uses the global document or window objects will not work correctly (for example custom popup menus),
they need to instead use the document/window of the popout. To get the document/window of the popout use the
following method on one of the elements rendered in the popout (for example a ref or target in an event handler):
- ```
- const currentDocument = this.selfRef.current.ownerDocument;
- const currentWindow = currentDocument.defaultView!;
- ```
In the above code selfRef is a React ref to the toplevel element in the tab being rendered.
Note: some libraries support popout windows by allowing you to specify the document to use,
for example see the getDocument() callback in agGrid at https://www.ag-grid.com/javascript-grid-callbacks/
Optional Props
Prop | Description |
---|---|
--------------- | ----------------- |
font | the |
icons | object |
onAction | function |
onRenderTab | function |
onRenderTabSet | function |
onModelChange | function |
onExternalDrag | function |
classNameMapper | function |
i18nMapper | function |
supportsPopout | if |
popoutURL | URL |
realtimeResize | boolean |
onTabDrag | function |
onRenderDragRect | callback |
onRenderFloatingTabPlaceholder | callback |
onContextMenu | callback |
onAuxMouseClick | callback |
onShowOverflowMenu | callback |
onTabSetPlaceHolder | callback |
iconFactory | a |
titleFactory | a |
Global Config attributes
Attributes allowed in the 'global' element
Attribute | Default | Description |
---|---|---|
------------- | |:-------------:| | |
splitterSize | 8 | width |
splitterExtra | 0 | additional |
legacyOverflowMenu | false | use |
enableEdgeDock | true | | |
tabEnableClose | true | allow |
tabCloseType | 1 | see |
tabEnableDrag | true | allow |
tabEnableRename | true | allow |
tabEnableFloat | false | enable |
tabClassName | null | | |
tabIcon | null | | |
tabEnableRenderOnDemand | true | whether |
tabDragSpeed | 0.3 | CSS |
tabBorderWidth | -1 | width |
tabBorderHeight | -1 | height |
tabSetEnableDeleteWhenEmpty | true | | |
tabSetEnableDrop | true | allow |
tabSetEnableDrag | true | allow |
tabSetEnableDivide | true | allow |
tabSetEnableMaximize | true | allow |
tabSetEnableClose | false | allow |
tabSetAutoSelectTab | true | whether |
tabSetClassNameTabStrip | null | height |
tabSetClassNameHeader | null | | |
tabSetEnableTabStrip | true | enable |
tabSetHeaderHeight | 0 | height |
tabSetTabStripHeight | 0 | height |
borderBarSize | 0 | size |
borderEnableAutoHide | false | hide |
borderEnableDrop | true | allow |
borderAutoSelectTabWhenOpen | true | whether |
borderAutoSelectTabWhenClosed | false | whether |
borderClassName | null | | |
borderSize | 200 | initial |
borderMinSize | 0 | minimum |
tabSetMinHeight | 0 | minimum |
tabSetMinWidth | 0 | minimum |
tabSetTabLocation | top | show |
rootOrientationVertical | false | the |
Row Attributes
Attributes allowed in nodes of type 'row'.
Attribute | Default | Description |
---|---|---|
------------- | |:-------------:| | |
type | row | | |
weight | 100 | | |
width | null | preferred |
height | null | preferred |
children | *required* | a |
Tab Attributes
Attributes allowed in nodes of type 'tab'.
Inherited defaults will take their value from the associated global attributes (see above).
Attribute | Default | Description |
---|---|---|
------------- | |:-------------:| | |
type | tab | | |
name | *required* | name |
altName | *optional* | if |
component | *required* | string |
config | null | a |
id | auto | | |
helpText | *optional* | An |
enableClose | *inherited* | allow |
closeType | *inherited* | see |
enableDrag | *inherited* | allow |
enableRename | *inherited* | allow |
enableFloat | *inherited* | enable |
floating | false | | |
className | *inherited* | | |
icon | *inherited* | | |
enableRenderOnDemand | *inherited* | whether |
borderWidth | *inherited* | width |
borderHeight | *inherited* | height |
Tab nodes have a getExtraData() method that initially returns an empty object, this is the place to
add extra data to a tab node that will not be saved.
TabSet Attributes
Attributes allowed in nodes of type 'tabset'.
Inherited defaults will take their value from the associated global attributes (see above).
Note: tabsets can be dynamically created as tabs are moved and deleted when all their tabs are removed (unless enableDeleteWhenEmpty is false).
Attribute | Default | Description |
---|---|---|
------------- | |:-------------:| | |
type | tabset | | |
weight | 100 | relative |
width | null | preferred |
height | null | preferred |
name | null | named |
config | null | a |
selected | 0 | index |
maximized | false | whether |
enableClose | false | allow |
id | auto | | |
children | *required* | a |
enableDeleteWhenEmpty | *inherited* | | |
enableDrop | *inherited* | allow |
enableDrag | *inherited* | allow |
enableDivide | *inherited* | allow |
enableMaximize | *inherited* | allow |
autoSelectTab | *inherited* | whether |
classNameTabStrip | *inherited* | | |
classNameHeader | *inherited* | | |
enableTabStrip | *inherited* | enable |
headerHeight | *inherited* | | |
tabStripHeight | *inherited* | height |
tabLocation | *inherited* | show |
minHeight | *inherited* | minimum |
minWidth | *inherited* | minimum |
Border Attributes
Attributes allowed in nodes of type 'border'.
Inherited defaults will take their value from the associated global attributes (see above).
Attribute | Default | Description |
---|---|---|
------------- | |:-------------:| | |
type | border | | |
size | *inherited* | size |
minSize | *inherited* | | |
selected | -1 | index |
id | auto | border_ |
config | null | a |
show | true | show/hide |
enableAutoHide | false | hide |
children | *required* | a |
barSize | *inherited* | size |
enableDrop | *inherited* | | |
autoSelectTabWhenOpen | *inherited* | whether |
autoSelectTabWhenClosed | *inherited* | whether |
className | *inherited* | | |
Model Actions
All changes to the model are applied through actions.
You can intercept actions resulting from GUI changes before they are applied by
implementing the onAction callback property of the Layout.
You can also apply actions directly using the Model.doAction() method.
This method takes a single argument, created by one of the following action
generators (typically accessed as `FlexLayout.Actions.Action | Description |
---|---|
------------- | -----| |
Actions.addNode(newNodeJson, | add |
Actions.moveNode(fromNodeId, | move |
Actions.deleteTab(tabNodeId) | delete |
Actions.renameTab(tabNodeId, | rename |
Actions.selectTab(tabNodeId) | select |
Actions.setActiveTabset(tabsetNodeId) | set |
Actions.adjustSplit(splitterNodeId, | adjust |
Actions.adjustBorderSplit(borderNodeId, | updates |
Actions.maximizeToggle(tabsetNodeId) | toggles |
Actions.updateModelAttributes(attributes) | updates |
Actions.updateNodeAttributes(nodeId, | updates |
Actions.floatTab(nodeId) | popout |
Actions.unFloatTab(nodeId) | restore |
Examples
- ``` js
- model.doAction(FlexLayout.Actions.updateModelAttributes({
- splitterSize:40,
- tabSetHeaderHeight:40,
- tabSetTabStripHeight:40
- }));
- ```
The above example would increase the size of the splitters, tabset headers and tabs, this could be used to make
adjusting the layout easier on a small device.
- ``` js
- model.doAction(FlexLayout.Actions.addNode(
- {type:"tab", component:"grid", name:"a grid", id:"5"},
- "1", FlexLayout.DockLocation.CENTER, 0));
- ```
This example adds a new grid component to the center of tabset with id "1" and at the 0'th tab position (use value -1 to add to the end of the tabs).
Note: you can get the id of a node (e.g., the node returned by the addNode
action) using the method node.getId().
If an id wasn't assigned when the node was created, then one will be created for you of the form `#Layout Component Methods to Create New Tabs
Methods on the Layout Component for adding tabs, the tabs are specified by their layout json.
Example:
- ```
- this.layoutRef.current.addTabToTabSet("NAVIGATION", {type:"tab", component:"grid", name:"a grid"});
- ```
This would add a new grid component to the tabset with id "NAVIGATION" (where this.layoutRef is a ref to the Layout element, see https://reactjs.org/docs/refs-and-the-dom.html ).
Layout | Description |
---|---|
------------- | -----| |
addTabToTabSet(tabsetId, | adds |
addTabToActiveTabSet(json) | adds |
addTabWithDragAndDrop(dragText, | adds |
addTabWithDragAndDropIndirect(dragText, | adds |
moveTabWithDragAndDrop( | Move |
Tab Node Events
You can handle events on nodes by adding a listener, this would typically be done
in the components constructor() method.
Example:
- ```
- constructor(props) {
- super(props);
- let config = this.props.node.getConfig();
- // save state in flexlayout node tree
- this.props.node.setEventListener("save", (p) => {
- config.subject = this.subject;
- };
- }
- ```
Event | parameters | Description |
---|---|---|
------------- | |:-------------:| | |
resize | | | |
close | | | |
visibility | | | |
save | | |
Running the Examples and Building the Project
First install dependencies:
- ```
- yarn install
- ```
Compile the project and run the examples:
- ```
- yarn start
- ```
Open your browser at http://localhost:8080/examples/ to show the examples directory, click on the examples to run them.
The 'yarn start' command will watch for changes to flexlayout and example source, so you can make changes to the code
and then refresh the browser to see the result.
To run the tests in the Cypress interactive runner use:
- ```
- yarn cypress
- ```
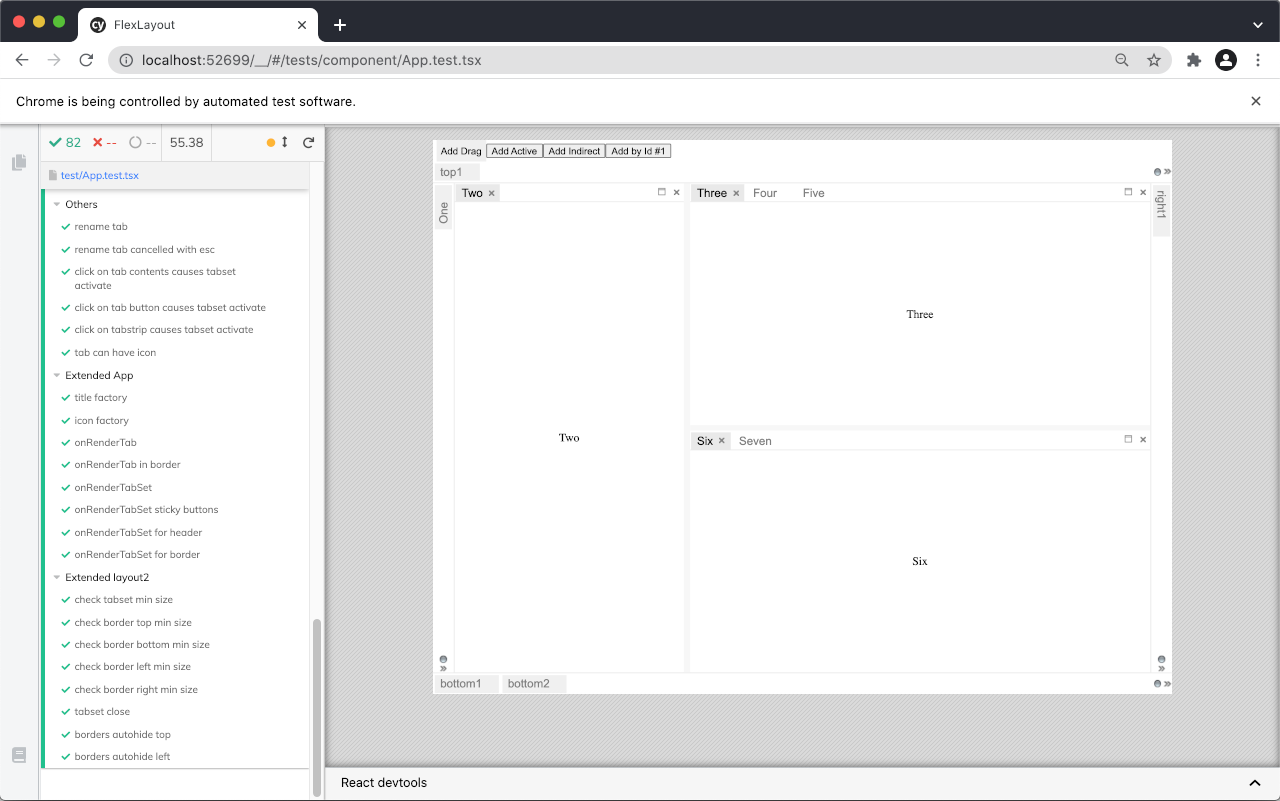
To build the npm distribution run 'yarn build', this will create the artifacts in the dist dir.
Alternative Layout Managers
Name | Repository |
---|---|
------------- | |:-------------| |
rc-dock | https://github.com/ticlo/rc-dock |
lumino | https://github.com/jupyterlab/lumino |
golden-layout | https://github.com/golden-layout/golden-layout |
react-mosaic | https://github.com/nomcopter/react-mosaic |