Moovie
Media player made for movies
README
Demo ▪️ Installation ▪️ Shortcuts ▪️ API ▪️ Integrations ▪️ Events
Styling ▪️ Plugins ▪️ Custom Events ▪️ i18n ▪️ Settings
◼️ Features:
-
- 🔧 Fully customizable and Easy-to-use
- 💎 Built-in caption offset adjust on the fly
- 🎬 Built-in support for .vtt and .srt caption files
- 🕹 Built-in Plugins, use the code that you really need!
- 🎯 Built-in CustomEvents, add multiple events that will run a specific currentTime
- 🖊 Add tracks/captions dynamically using our API
- 🗃 Add tracks/captions locally on the fly (no server or upload required)
- 🌠 Adjust speed on the fly
- 🛠 Standardized events / shortcuts / API
- 🖌 Caption customization
- 💪 No dependencies, built with VanillaJS
- 🌎 Tested in all modern browsers
- 💻 Responsive
- 🗃 Integration with webtorrent.js, dash.js, Shaka Player and hls.js
- 🌎 Internationalization (i18n) of controls
◼️ Demo:
-
https://mooviejs.com/
◼️ Installation (Browser):
-
- ``` js
- <script src="path/to/moovie.js"></script>
- ```
- ``` js
- <link rel="stylesheet" href="path/to/moovie.css">
- ```
- ``` html
- <video id="example" poster="<<path-to-poster>"
- <source src="<<path-to-file.mp4>" type="video/mp4"
- <track kind="captions" label="Portuguese" srclang="pt" src="<<path-to-caption.vtt>"
- <track kind="captions" label="English" srclang="en" src="<<path-to-caption.vtt>"
- Your browser does not support the video tag.
- </video>
- ```
- ``` js
- document.addEventListener("DOMContentLoaded", function() {
- var demo = new Moovie({
- selector: "#example",
- dimensions: {
- width: "100%"
- }
- });
- });
- ```
Note: Do not forget to include "icons" folder.
◼️ Installation (NPM):
-
1 - Install npm package- ``` js
- npm i mooviejs
- ```
- ``` js
- <link rel="stylesheet" href="path/to/moovie.css">
- ```
- ``` html
- <video id="example" poster="<<path-to-poster>"
- <source src="<<path-to-file.mp4>" type="video/mp4"
- <track kind="captions" label="Portuguese" srclang="pt" src="<<path-to-caption.vtt>"
- <track kind="captions" label="English" srclang="en" src="<<path-to-caption.vtt>"
- Your browser does not support the video tag.
- </video>
- ```
- ``` js
- const Moovie = require('mooviejs');
- var demo = new Moovie({
- selector: "#example",
- dimensions: {
- width: "100%"
- },
- icons: {
- path: "<path/to/icons/folder>"
- }
- });
- ```
◼️ CDN:
-
You can use our CDN (provided by JSDelivr) for the JavaScript and CSS files.
- ``` html
- // Javascript
- <script src="https://cdn.jsdelivr.net/gh/BMSVieira/moovie.js@latest/js/moovie.min.js"></script>
- // CSS
- <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/BMSVieira/moovie.js@latest/css/moovie.min.css">
- ```
◼️ Captions:
-
Currently it has full support for WebVTT(.vtt) and SubRip(.srt).
▪️ Dynamically (Public server required)
Use the standard html as the example below (source must be in a public server with cross-origin headers).
- ``` html
- <video>
- <track kind="captions" label="<<Language>" srclang="<SourceLang>" src="<path-to-caption.vtt/.srt>"
- </video
- ```
▪️ Locally (No server or upload required)
- ``` html
- Video Player ➔ ⚙️ ➔ Captions ➔ Load Locally
- ```
◼️ Caption Offset Adjust:
-
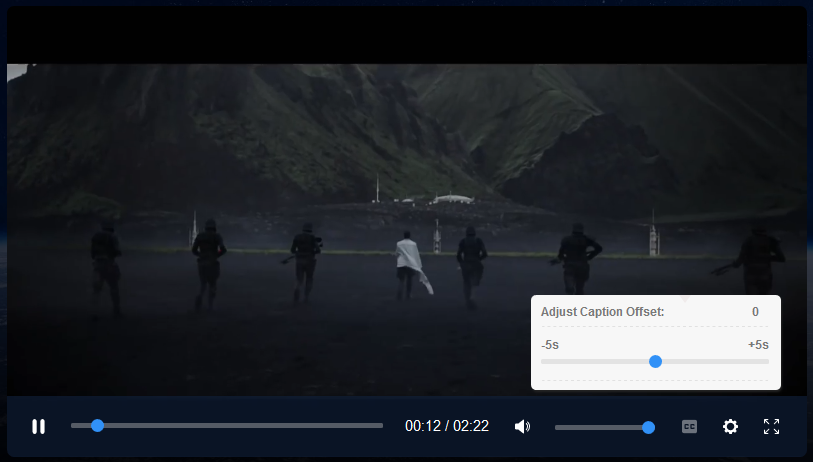
◼️ Shortcuts:
-
A player will bind the following keyboard shortcuts when it has focus.
Key | Description |
---|---|
--- | --- |
`SpaceBar` | Toggle |
`K` | Toggle |
`F` | Toggle |
`C` | Toggle |
`M` | Toggle |
`ArrowRight` | Forward |
`ArrowLeft` | Backward |
`Shift`+`W`| | |
`Shift`+`S`| |
◼️ API > Methods:
-
Play/Pause video
- ``` js
- demo.togglePlay();
- ```
Enable/Disable subtitles
- ``` js
- demo.toggleSubtitles();
- ```
Enable/Disable fullscreen
- ``` js
- demo.toggleFullscreen();
- ```
Remove current player and unbinds all its events
- ``` js
- demo.destroy();
- ```
Build a new player
- ``` js
- demo.build();
- ```
Add multiple/single captions to player
Name | Type | Default | Description |
---|---|---|---|
--- | --- | |--- | --- |
`label` | `string` | `New | Name |
`srclang` | `string` | `New` | Country |
`src` | `string` | `---` | Path |
- ``` js
- demo.addTrack({
- options : {
- 0: {
- label: 'Italian',
- srclang: "it",
- src: "<<path-to-file.vtt/.srt"
- },
- 1: {
- label: 'Spanish',
- srclang: "es",
- src: "<<path-to-file.vtt/.srt"
- }
- },
- onComplete: function(){
- console.log("Completed!");
- }
- });
- ```
Apply changes to current player.
Name | Type | Description |
---|---|---|
--- | --- | --- |
`video` | `string` | New |
`video` | `string` | New |
`captions` | `boolean` | Remove |
- ``` js
- demo.change({
- video: {
- videoSrc: "<<path-to-video>>",
- posterSrc: "<<path-to-poster>>"
- },
- captions:{
- clearCaptions: true
- },
- onComplete: function(){
- console.log("Completed!");
- }
- });
- ```
◼️ API > Gets:
-
- ``` js
- // Returns player DOM element
- demo.playerElement
- // Returns a boolean indicating if the current player is playing.
- demo.playing
- // Returns a boolean indicating if the current player is paused.
- demo.paused
- // Returns a boolean indicating if the current player is stopped.
- demo.stopped
- // Returns a boolean indicating if the current player has finished playback.
- demo.ended
- // Returns currentTime of the player.
- demo.currentTime
- // Returns the duration for the current media.
- demo.duration
- // Returns a boolean indicating if the current player is seeking.
- demo.seeking
- // Returns the volume of the player.
- demo.volume
- // Returns a boolean indicating if the current player is muted.
- demo.muted
- // Returns current playRate
- demo.speed
- // Returns mininum speed allowed
- demo.minimumSpeed
- // Returns maximum speed allowed
- demo.maximumSpeed
- // Returns mininum caption offset allowed
- demo.minimumOffset
- // Returns maximum caption offset allowed
- demo.maximumOffset
- // Returns current caption offset
- demo.captionOffset
- // Returns current source of the player
- demo.source
- ```
◼️ API > Sets:
-
- ``` js
- // Set currentTime to given number(seconds)
- demo.currentTime = 60
- // Set player's volume to given number (0.5 is half the volume)
- demo.volume = 0.5
- // Set player's playbackRate to given number (0.1 to 8)
- demo.speed = 2
- // Set player's caption offset to given number (-5 to 5)
- demo.captionOffset = 2
- // Mute or Unmute player (boolean)
- demo.muted = true
- ```
◼️ Integrations:
-
| | Name & Info | Example |
| :-: | :-: | --- |
|
For more info read WebTorrent documentation.
| [Codepen](https://codepen.io/BMSVieira/pen/vYgpvJX)||

For more info read dash.js documentation. | [Codepen](https://codepen.io/BMSVieira/pen/BapJqBV)||

For more info read Shaka Player documentation. | [Codepen](https://codepen.io/BMSVieira/pen/PoWEVwe)||

For more info read hls.js documentation. | [Codepen](https://codepen.io/BMSVieira/pen/ExZorLW)|
◼️ Events:
-
- ``` js
- demo.video.addEventListener("canplay", (event) => {
- console.log(event);
- });
- ```
Event | Description |
---|---|
--- | --- |
`abort`| | |
`canplay`| | |
`canplaythrough`| | |
`durationchange`| | |
`emptied`| | |
`error`| | |
`interruptbegin`| | |
`interruptend`| | |
`loadeddata`| | |
`loadedmetadata`| | |
`loadstart`| | |
`pause`| | |
`play`| | |
`playing`| | |
`progress`| | |
`ratechange`| | |
`seeked`| | |
`seeking`| | |
`stalled`| | |
`suspend`| | |
`timeupdate`| | |
`volumechange`| | |
`waiting`| | |
`captionchange` | Sent |
`offsetchange`| | |
`togglecaption`| |
◼️ Styling > Colors:
-
Check below a list of variables and what they are used for:
Name | Description | Default |
---|---|---|
--- | --- | --- |
`--moovie_main_color` | Moovie | |
`--moovie_bg_controls` | Background | `rgba(16, |
`--moovie_bg_submenu` | Submenu | |
`--moovie_bg_cuetimer` | Cuetimer | |
`--moovie_submenu_options_fcolor`| | ||
`--moovie_topic_submenu_fcolor` | Submenu | |
`--moovie_currenttime_color` | CurrentTime | `white`| |
`--moovie_submenu_options_fsize` | Submenu | `10pt`| |
`--moovie_topic_submenu_fsize` | Submenu | `9pt`| |
`--moovie_currenttime_fsize`| | `11pt`| | |
`--moovie_cuetimer_fsize`| | `9pt`| | |
`--moovie_svgicons_width` | Icons | `15px`| |
`--moovie_padding_controls`| | `13px`| | |
`--moovie_caption_fcolor`| | `white`| | |
`--moovie_cuetimer_fcolor`| | `white`| |
◼️ Styling > Icons:
-
moovie uses .svg icons that are stored in the icons folder that is located by default in the root, however, you can specify a new location in the config options.
- ``` js
- config: {
- icons: {
- path: "./path/to/folder/"
- }
- }
- ```
Folder structure:
- ``` html
- moovie/
- ├── icons/
- │ ├── back.svg
- │ ├── caption.svg
- │ ├── cc.svg
- │ ├── cog.svg
- │ ├── fullscreen.svg
- │ ├── mute.svg
- │ ├── next.svg
- │ ├── pause.svg
- │ ├── play.svg
- │ ├── volume.svg
- |
- ├── css/
- │ ├── moovie.css
- |
- ├── js/
- │ ├── moovie.js
- ```
◼️ Plugins:
-
There are external plugins you can use to add extra features.
to call a plugin, follow the example below:
- ``` html
- <script src="path/to/plugin.js"></script>
- ```
- ``` js
- // Initialize Moovie
- var demo = new Moovie({selector: "#example"});
- // Call External Plugin (Playlist example)
- var PlaylistPlugin = new _Moovie_Playlist({reference: demo});
- ```
List of available plugins:
Name | Description | | |
---|---|---|
--- | --- | --- |
`_Moovie_Playlist` | Create | [More |
◼️ Custom Events:
-
You can add multiple events that will run at a specific currentTime, for example:
- ``` js
- var demo = new Moovie({
- selector: "#example",
- customEvents: [
- {
- type: "skip",
- to: "56",
- starttime: "10",
- endtime: "20",
- content: "Skip Intro",
- position: "bottom-left",
- class: "my_class"
- },
- {
- ...
- }
- ]
- });
- ```
Option | Parameters | Description |
---|---|---|
--- | --- | --- |
`type` | `skip`, | Type |
`to` | `int`, | Parameters |
`starttime` | `int`| | |
`endtime` | `int`| | |
`content` | `string`| | |
`position` | `top-left`, | Button's |
`class` | `string` | Custom |
◼️ i18n Support:
-
You can translate the player to any language you want in the configurations.
- ``` js
- config: {
- i18n : {
- play : "(Play:Pause)",
- mute : "(Mute:Unmute)",
- subtitles : "(Enable:Disable) Subtitles",
- ...
- }
- }
- ```
How translation works is very simple, all text between brackets are the "changing" parts of the string, and in the left side of the ":" is the default state string.
- ``` js
- ([DEFAULT STATE]:[AFTER ACTION STATE]) [CONTINUES STRING]
- ```
Working examples:
- ``` js
- // English
- subtitles : "(Enable:Disable) Subtitles",
- // Portuguese
- subtitles : "(Ativar:Desativar) Legendas",
- // French
- subtitles: "(Activer:Désactiver) Les sous-titres"
- ```
Option | Default | Description |
---|---|---|
--- | :-: | --- |
`play` | `(Play:Pause)` | Play |
`mute` | `(Mutar:Desmutar)` | Mute |
`subtitles` | `(Enable:Disable) | Subtitles |
`config` | `Settings` | Settings |
`fullscreen` | `(Enter:Exit) | Fullscreen |
`main_topic` | `settings:` | Submenu's |
`main_caption` | `Captions` | Submenu's |
`main_offset` | `Caption | Submenu's |
`main_speed` | `Speed` | |Submenu's |
`main_disabled` | `Disabled` | Submenu's |
`main_default` | `Default` | Submenu's |
`caption_topic` | `Captions:` | Caption's |
`caption_back` | `Back` | Caption's |
`caption_load` | `Load | Caption's |
`offset_topic` | `Adjust | Offset's |
`speed_topic` | `Speed | Speed's |
◼️ Settings:
-
Option | Type | Description |
---|---|---|
--- | --- | --- |
`selector` | `String` | Specify |
◼️ | | | |
`width` | `string` | Width |
◼️ | | | |
`captionOffset`| | Indicates | |
`playrateSpeed`| | Indicates | |
`captionSize`| | Indicates | |
◼️ | | | |
`playtime`| | Indicates | |
`mute`| | Indicates | |
`volume`| | Indicates | |
`subtitles`| | Indicates | |
`config`| | Indicates | |
`fullscreen`| | Indicates | |
`submenuCaptions`| | Indicates | |
`submenuOffset`| | Indicates | |
`submenuSpeed`| | Indicates | |
`allowLocalSubtitles`| | Indicates | |
◼️ | | | |
`path`| | Specify |
- ``` js
- document.addEventListener("DOMContentLoaded", function() {
- var demo = new Moovie({
- selector: "#example",
- dimensions: {
- width: "100%"
- },
- config: {
- storage: {
- captionOffset: false,
- playrateSpeed: false,
- captionSize: false
- },
- controls : {
- playtime : true,
- mute : true,
- volume : true,
- subtitles : true,
- config : true,
- fullscreen : true,
- submenuCaptions : true,
- submenuOffset : true,
- submenuSpeed : true,
- allowLocalSubtitles : true
- },
- i18n : {
- play : "(Play:Pause)",
- mute : "(Mute:Unmute)",
- subtitles : "(Enable:Disable) Subtitles",
- config : "Settings",
- fullscreen : "(Enter:Exit) Fullscreen",
- main_topic: "settings:",
- main_caption: "Captions",
- main_offset: "Caption Offset",
- main_speed: "Speed",
- main_disabled: "Disabled",
- main_default: "Default",
- caption_topic: "Captions:",
- caption_back: "Back",
- caption_load: "Load Locally",
- offset_topic: "Adjust Caption Offset",
- speed_topic: "Speed Adjust"
- }
- },
- icons: {
- path: "./path/to/folder/"
- }
- });
- });
- ```
◼️ Sponsors:
-
