README
XO
JavaScript/TypeScript linter (ESLint wrapper) with great defaults
Opinionated but configurable ESLint wrapper with lots of goodies included. Enforces strict and readable code. Never discuss code style on a pull request again! No decision-making. No .eslintrcto manage. It just works!
It uses ESLint underneath, so issues regarding built-in rules should be opened over there .
XO requires your project to be ESM .
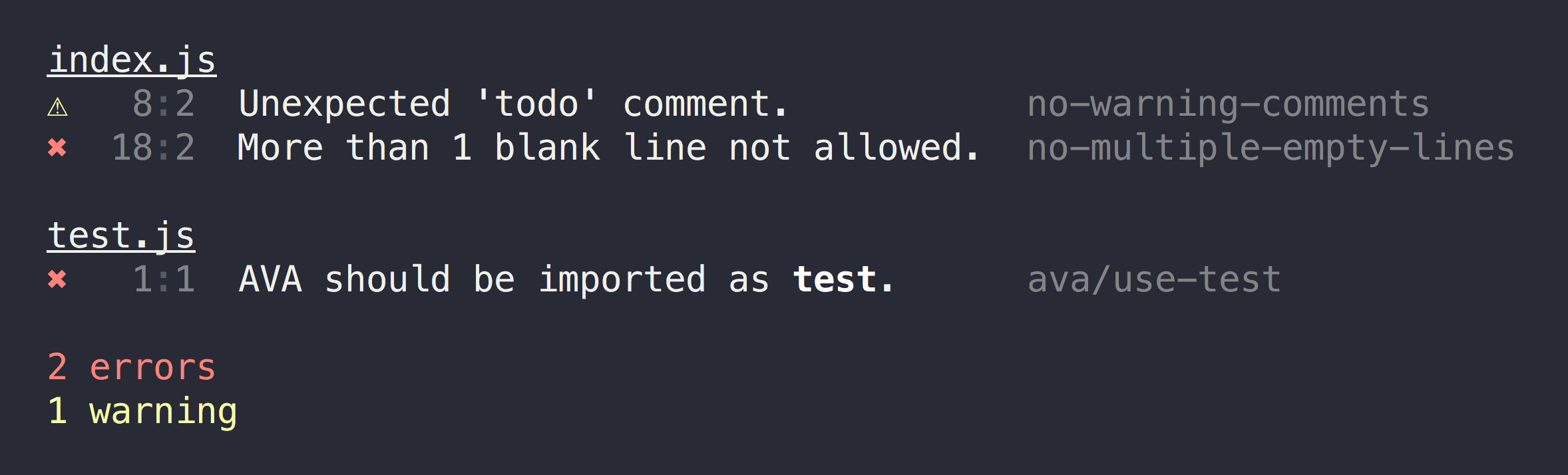
Highlights
Beautiful output.
Zero-config, but configurable when needed .
Enforces readable code, because you read more code than you write.
No need to specify file paths to lint as it lints all JS/TS files except for commonly ignored paths .
Config overrides per files/globs.
TypeScript supported by default.
Includes many useful ESLint plugins, like unicorn , import , ava , n and more.
Automatically enables rules based on the engines field in your package.json.
Caches results between runs for much better performance.
Super simple to add XO to a project with $ npm init xo .
Fix many issues automagically with $ xo --fix.
Open all files with errors at the correct line in your editor with $ xo --open.
Specify indent and semicolon preferences easily without messing with the rule config.
Optionally use the Prettier code style.
Great editor plugins .
Install
- ``` shell
- npm install xo --save-dev
- ```
You must install XO locally. You can run it directly with $ npx xo.
JSX is supported by default, but you'll need eslint-config-xo-react for React specific linting. Vue components are not supported by default. You'll need eslint-config-xo-vue for specific linting in a Vue app.
Usage
- ``` null
- $ xo --help
- Usage
- $ xo [<file|glob> ...]
- Options
- --fix Automagically fix issues
- --reporter Reporter to use
- --env Environment preset [Can be set multiple times]
- --global Global variable [Can be set multiple times]
- --ignore Additional paths to ignore [Can be set multiple times]
- --space Use space indent instead of tabs [Default: 2]
- --no-semicolon Prevent use of semicolons
- --prettier Conform to Prettier code style
- --node-version Range of Node.js version to support
- --plugin Include third-party plugins [Can be set multiple times]
- --extend Extend defaults with a custom config [Can be set multiple times]
- --open Open files with issues in your editor
- --quiet Show only errors and no warnings
- --extension Additional extension to lint [Can be set multiple times]
- --cwd=<dir> Working directory for files
- --stdin Validate/fix code from stdin
- --stdin-filename Specify a filename for the --stdin option
- --print-config Print the ESLint configuration for the given file
- Examples
- $ xo
- $ xo index.js
- $ xo *.js !foo.js
- $ xo --space
- $ xo --env=node --env=mocha
- $ xo --plugin=react
- $ xo --plugin=html --extension=html
- $ echo 'const x=true' | xo --stdin --fix
- $ xo --print-config=index.js
- Tips
- - Add XO to your project with `npm init xo`.
- - Put options in package.json instead of using flags so other tools can read it.
- ```
Default code style
Any of these can be overridden if necessary.
Tab indentation (or space)
Semicolons (or not)
Single-quotes
Trailing comma for multiline statements
No unused variables
Space after keyword if (condition) {}
Always ===instead of ==
Check out an example and the ESLint rules .
Workflow
The recommended workflow is to add XO locally to your project and run it with the tests.
Simply run $ npm init xo(with any options) to add XO to your package.json or create one.
Before/after
- ``` diff
- {
- "name": "awesome-package",
- "scripts": {
- - "test": "ava",
- + "test": "xo && ava"
- },
- "devDependencies": {
- - "ava": "^3.0.0"
- + "ava": "^3.0.0",
- + "xo": "^0.41.0"
- }
- }
- ```
Then just run $ npm testand XO will be run before your tests.
Config
You can configure XO options with one of the following files:
As JSON in the xoproperty in package.json:
- ``` json
- {
- "name": "awesome-package",
- "xo": {
- "space": true
- }
- }
- ```
As JSON in .xo-configor .xo-config.json:
- ``` json
- {
- "space": true
- }
- ```
As a JavaScript module in .xo-config.jsor xo.config.js:
- ``` js
- module.exports = {
- space: true
- };
- ```
For ECMAScript module (ESM) packages with "type": "module" , as a JavaScript module in .xo-config.cjsor xo.config.cjs:
- ``` js
- module.exports = {
- space: true
- };
- ```
Globals and rules can be configured inline in files.