Oxc
A suite of high-performance tools for JavaScript and TypeScript written in ...
README
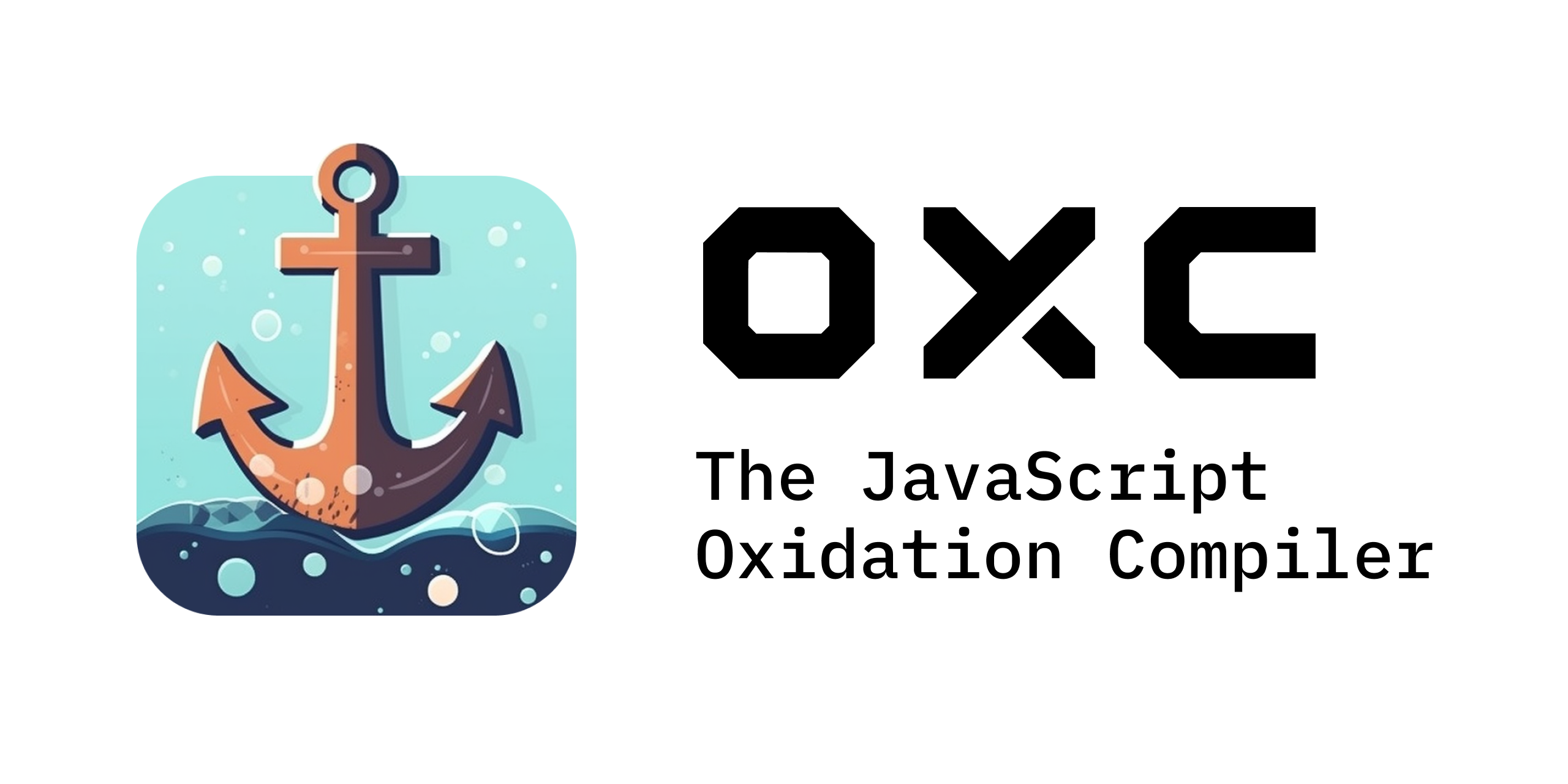
⚓ Oxc
The Oxidation Compiler is creating a suite of high-performance tools for JavaScript and TypeScript.
Oxc is building a parser, linter, formatter, transpiler, minifier, resolver ... all written in Rust.
💡 Philosophy
This project shares the same philosophies as [Biome][biome] and [Ruff][ruff].
1. JavaScript tooling could be rewritten in a more performant language.
2. An integrated toolchain can tap into efficiencies that are not available to a disparate set of tools.
⚡️ Quick Start
The linter is ready to catch mistakes for you. It comes with over 60 default rules and no configuration is required.
To start using, install [oxlint][npm-oxlint] or via npx:
- ```bash
- npx oxlint@latest
- ```
To give you an idea of its capabilities, here is an example from the [vscode] repository, which finishes linting 4000+ files in 0.5 seconds.
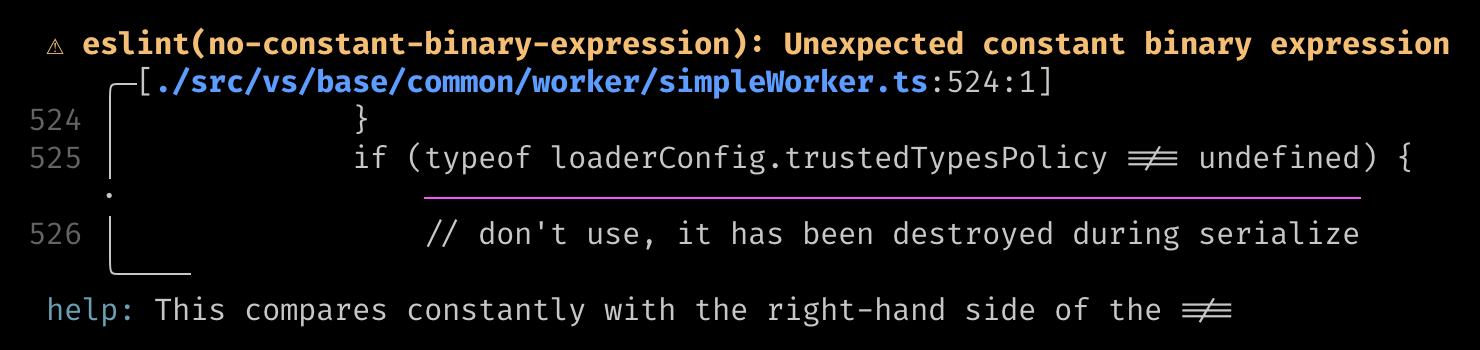
⚡️ Performance
The parser aim to be the fastest Rust-based ready-for-production parser.
The linter is more than 50 times faster than [ESLint], and scales with the number of CPU cores.
⌨️ Programming Usage
Rust
Individual crates are published, you may use them to build your own JavaScript tools.
The umbrella crate oxc exports all public crates from this repository.
The AST and parser crates oxc_ast and oxc_parse are production ready.
See crates/*/examples for example usage.
While Rust has gained a reputation for its comparatively slower compilation speed,
we have dedicated significant effort to fine-tune the Rust compilation speed.
Our aim is to minimize any impact on your development workflow,
ensuring that developing your own Oxc based tools remains a smooth and efficient experience.
This is demonstrated by our CI runs,
where warm runs complete in 5 minutes.
Node.js
You may use the parser via napi: [oxc-parser][npm-napi]
🔸 AST and Parser
Oxc maintains its own AST and parser, which is by far the fastest and most conformant JavaScript and TypeScript (including JSX and TSX) parser written in Rust.
As the parser often represents a key performance bottleneck in JavaScript tooling,
any minor improvements can have a cascading effect on our downstream tools.
By developing our parser, we have the opportunity to explore and implement well-researched performance techniques.
While many existing JavaScript tools rely on [estree] as their AST specification,
a notable drawback is its abundance of ambiguous nodes.
This ambiguity often leads to confusion during development with [estree].
The Oxc AST differs slightly from the [estree] AST by removing ambiguous nodes and introducing distinct types.
For example, instead of using a generic [estree] Identifier,
the Oxc AST provides specific types such as BindingIdentifier, IdentifierReference, and IdentifierName.
This clear distinction greatly enhances the development experience by aligning more closely with the ECMAScript specification.
🏆 Parser Performance
Our [benchmark][parser-benchmark] reveals that the Oxc parser surpasses the speed of the [swc] parser by approximately 2 times and the [Biome] parser by 3 times.
How is it so fast?
- AST is allocated in a memory arena (bumpalo) for fast AST memory allocation and deallocation.
- Short strings are inlined by CompactString.
- No other heap allocations are done except the above two.
- Scope binding, symbol resolution and some syntax errors are not done in the parser, they are delegated to the semantic analyzer.
🔸 Linter
The linter embraces convention over configuration, eliminating the need for extensive configuration and plugin setup.
Unlike other linters like [ESLint], which often require intricate configurations and plugin installations (e.g. [@typescript-eslint]),
our linter only requires a single command that you can immediately run on your codebase:
- ```bash
- npx oxlint@latest
- ```
We also plan to port essential plugins such as [eslint-plugin-import] and [eslint-plugin-jest].
🏆 Linter Performance
The linter is 50 - 100 times faster than [ESLint] depending on the number of rules and number of CPU cores used.
It completes in less than a second for most codebases with a few hundred files and completes in a few seconds for
larger monorepos. See bench-javascript-linter for details.
As an upside, the binary is approximately 3MB, whereas [ESLint] and its associated plugin dependencies can easily exceed 100.
You may also download the linter binary from the latest release tag as a standalone binary,
this lets you run the linter without a Node.js installation in your CI.